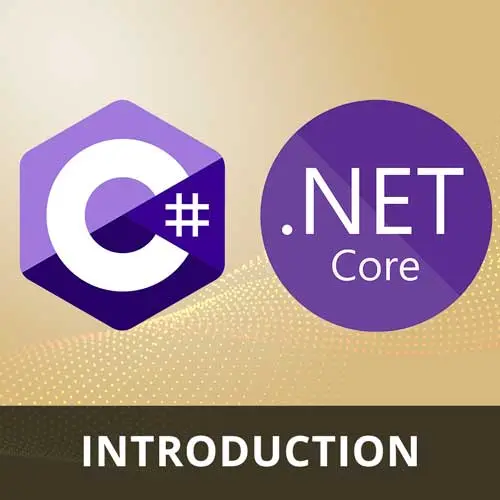
Check out a free preview of the full C# and .NET Basics course
The "Lists" Lesson is part of the full, C# and .NET Basics course featured in this preview video. Here's what you'd learn in this lesson:
Spencer discusses lists which are a common class for collections in .NET that provide flexibility in creating lists of specific types. He covers methods such as add, remove, and add range that can be used with lists. He also briefly mentions other collection types like hash set, dictionary, immutable array, queue, and stack.
Transcript from the "Lists" Lesson
[00:00:00]
>> Spencer Schneidenbach: So let's talk about what I think that is the favorite collection type of a .NET developer, the list. The list is ubiquitous in .NET collections. Let me show you what it looks like. So we talked about boxes of strings and boxes of int, but in reality, you probably wouldn't define code like this.
[00:00:17]
This is no better than setting a variable. But you would, and you often do, define a list of strings or a list of integers. And you could say, I wanna add things to this list. I wanna add Spencer to this list, bring him on the bus. In fact, let's name this bus.
[00:00:35]
I wanna bring him on the bus. Let's bring him on the bus. George wants to go on the bus. Let's bring George. Can int go on the bus? Int can't go on the bus. Compiler won't let int go on the bus. Which is right, right? This is the most common class for collections in .NET in terms of the use by developers, this and array, but mainly list people love to materialize things as lists for some reason.
[00:01:05]
This is good, because you get the flexibility of a generic type, so you can create lists of strings or lists of other objects, and then prevent other objects from joining in on the party. We want Spencer and George on the bus. We don't want integers on the bus.
[00:01:18]
You can also define lists ahead of time, same as you can arrays using what's called collection initializer syntax in newer versions of C sharp, but this is the way that they've kind of been defined in previous years with curly braces and using the lists constructor. So there's a few methods on list.
[00:01:42]
There's actually a bunch, but the ones that you need to know about are Add, Remove, RemoveAt, AddRange, which will allow you to add a collection of strings, add range. So we would add Mary and Ann, right? So that would give you an IEnumerable of string that would add two more elements to that array or sorry to that list.
[00:02:06]
And under the hood, a list just takes an array and just has a fixed amount of elements in it, and then resizes it when it needs to. So pretty cool. You can browse the source code yourself. There's not a lot of magic behind it. It's just .NET making things as convenient as it can for you.
[00:02:23]
Couple of things you should know about lists. There's a for each method on the list. Do not use it, and the reason that you shouldn't use it is because it shouldn't be there. [LAUGH] In my strong opinion, this, to me, is just not a way there's a construct in the language for doing ForEach and it is called the ForEach loop, it is more ubiquitous.
[00:02:44]
Are you gonna break the universe if you do this? No, it's just not a good habit to do so. And Microsoft has said just you shouldn't use this. And list is very powerful and it is versatile, but .NET devs love to use list sometimes to the point of absurdity especially when you're defining a method that has a specific behavior and you need to operate on a collection of objects.
[00:03:07]
And that developer decides that that collection of objects must be a list. So, it must be a list ahead of time. It might have probably been more suitable to define that method to take in IEnumerable instead. That would have probably been more practical. There's a couple of other collection types, the most important one, we already talked about array, the hash set is like a list, but you can't add duplicates to it.
[00:03:32]
So if you go here, you can't add two spencers to the bus. You also notice that it does not have AddRange. You can only add one thing at a time, little bit of a quirk, but what this will inevitably do is we'll just skip adding this, and it will just end up with a collection of one it will silently not work.
[00:03:52]
So it will continue executing. Dictionary is probably the one that I use the most, next to array list and IEnumerable, dictionary is my heart and soul and that basically allows you to say, I declare it as dict and then I say new dictionary and then I say I just want a string and I wanna be able to look up integers with strings.
[00:04:18]
So what I can do is, I can set the value of this dictionary. So I can say that if I'm looking up the element at section asdf, I want to get the value of three, but I'm using them with, just very basic types. You can use these with classes as well, right?
[00:04:35]
So you get a lot of flexibility. Dictionary is one of the collections that I use to usually sometimes track state or or make sure that if I have a value and I need to cache it, I might cache it very quickly inside of a dictionary and then look it up later to see if it's there.
[00:04:53]
And if it's not, I'll add it so I can refer back to it, right? You don't want to look up the same record 1000 times when you can just look it up once in a loop. So a dictionary is a good construct to do that in. There are a few others.
[00:05:07]
Immutable array is one that I use a ton as well. I will often on most collection methods, actually all of them that implement IEnumerable, there is a ToList method. So you can materialize that to a list, it's called materializing. It will create a new other list or in this case another bus.
[00:05:28]
I should call this var. Other bus that will also create a list. You could also do ToArray and that will create an array. You can also do ToImmutableArray which is something that I do a lot because oftentimes you can mutate arrays, you can mutate lists. I believe in immutable objects because it keeps my programming consistent.
[00:05:49]
I want to make sure that people can't edit the things that I'm creating a lot of the times. So I'll create an immutable array so that way they can't actually modify contents of that array. So, a couple of others, Queue and stack, which are last in first out and first in first out collections I, in practice, almost never use them, but I know that they exist in case I ever do, in case I ever need that kind of construct.
[00:06:15]
So yeah, here's my spicy opinion. I use immutable array a ton, I use dictionary a lot, and if I need to add or remove elements, I'll use a list most of the time.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops