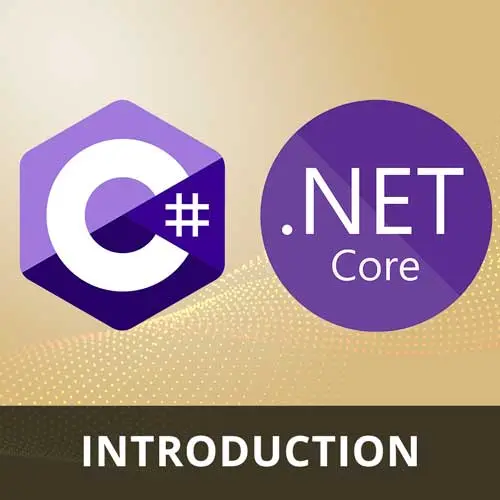
Check out a free preview of the full C# and .NET Basics course
The "Introduction to LINQ" Lesson is part of the full, C# and .NET Basics course featured in this preview video. Here's what you'd learn in this lesson:
Spencer introduces the concept of LINQ (Language Integrated Query) in .NET. He explains how LINQ allows for easy querying and manipulation of collections of objects. He also discusses the lazy evaluation nature of LINQ and provides examples of how to materialize the results.
Transcript from the "Introduction to LINQ" Lesson
[00:00:00]
>> Spencer Schneidenbach: Let's go next. So LINQ, Language Integrated Query is probably one of the defining features of .Net, this is one of the features that makes .Net so easy to love. Somebody asked about JavaScript and MapReduce filter. This is C#'s implementation of that, so let's talk about it. Imagine that you have an employee object, as we have had many times, or a person object.
[00:00:25]
And you need to get names of all the employees who are earning over $100,000, and you need to display these names on the page, sort in order. So you could do something like this, where you define a list of employees, where you get your employees from your repository, for instance.
[00:00:38]
And then you start tracking your highEarners in a separate list, and you spin through and you foreach through each of the employees. And you say if the employee salary is greater than 100,000, well, then add them to the highEarners list, right? We wanna keep track of them separately.
[00:00:51]
We're trying to get those, boss has asked for a report. And then we wanna sort that list. So there is a sort function inside of the list of t construct. It allows you to sort those two objects, so that way you're able to say, how do you want this list to be ordered?
[00:01:06]
And then you can spin through and go highEarner in highEarners, and then in console write name, and then show those names or print on a report or whatever. With LINQ, you're able to do that very naturally using a very kinda natural syntax. So this is what LINQ looks like.
[00:01:26]
You can do the exact same thing in way less code, but way clearer in a way that like this, this is difficult, it's possible, but it's a little more difficult to understand. Whereas this, it's kind of just I can see where salary is greater than a 100,000. I want you to order by name, and I want you to select all of these elements from this array.
[00:01:50]
This is really where the rubber meets the road when it comes to Lambda functions. The thing that we just talked about. Lambda functions are used by LINQ as a way of saying, hey, I need you to give me a function that tells me whether or not this salary is over 100,000.
[00:02:04]
I need you to tell me what you want me to order by. So you want me to order by name? I want you to get it here. And then I just need the names of all those employees. I don't need to know anything else about them. I wanna save memory, I wanna save space on my piece of paper.
[00:02:16]
So just print the names to console or print the names to the piece of paper. That's the only thing that I need. That's LINQ, it allows you to really powerfully operate on collections in a really kind of intuitive way, and it gets easy as you start typing and doing it.
[00:02:31]
I'm gonna talk about one major syntax for LINQ, the one that I see in the real world, which is the Lambda syntax. There is a different syntax that looks very similar to SQL. I will tell you that in practice, this is never used. I never see anybody write this like this, but when LINQ was originally created, they had a query syntax, God bless Microsoft's heart, nobody uses it.
[00:02:58]
>> Spencer Schneidenbach: So why did we talk about all those things ahead of all of this? Well, the technologies that make LINQ possible are generics, IEnumerable of T, extension methods. All of these things that you see, those don't exist on those collections, they're extension methods on those collection types, right, and Lambda expressions.
[00:03:17]
So we've kind of combined all of that in order to access our collections and transform them and do things with them, then in a really powerful way. So one kind of interesting property of LINQ is we talked about, remember how I said you need to put a pin in IEnumerable, and how they're lazily evaluated.
[00:03:36]
One of the cool things about LINQ and one of the slightly confusing things about LINQ is that when you declare this highEarners. At no point until you start assessing members of this collection do these methods actually go through and actually start comparing salaries and ordering by name. Which is a little different.
[00:03:56]
When you do map, or reduce, or filter in JavaScript, those operations happen immediately, right? In C#, that's not the case, it returns, it's what's called lazily evaluated. So when you declare it at that point, you're not enumerating through it. Only when you actually start spinning through it, do you actually start accessing and do all of these things start running, which is really interesting.
[00:04:26]
It's a small kind of important property to know, it's something to put in the back of your head. As you're going through your C# journey, you'll experience situations where you become very aware of this, but going on it's important to know it. I mentioned to ToList and ToArray, that's the easiest way to take a lazy evaluation and kick it into high gear.
[00:04:48]
It's called eagerly evaluated at that point. And that's typically what developers use to quote unquote, materialize a list. It will actually go through and run those where and select methods. I already talked about query syntax versus Lambda syntax and that we're gonna focus on using Lambda syntax exclusively.
[00:05:08]
I am going to combine as many of these sections as I can and just kind of show some code examples of using LINQ and things that I think you need to look out for when you're using it.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops