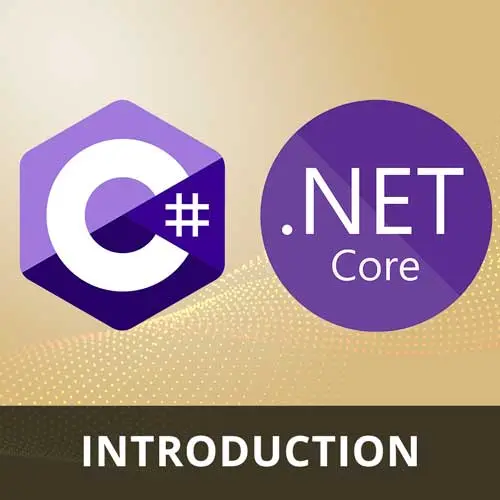
Lesson Description
The "Inheritance" Lesson is part of the full, C# and .NET Basics course featured in this preview video. Here's what you'd learn in this lesson:
Spencer explains that objects can inherit properties and behaviors from other objects, and demonstrates how to declare a class that inherits from another class. He also demonstrates adding accessibility modifiers such as protected, private, and public, and shows how they can be used to control access to methods and properties within a class hierarchy.
Transcript from the "Inheritance" Lesson
[00:00:00]
>> Spencer Schneidenbach: Nice little introduction to records and classes. Boom, let's move on to inheritance. This is probably, I'd say, one of the, besides classes, another really important kinda thing to understand about object-oriented languages and C Sharp. First of all, I've mentioned that all objects inherit from object. So if I declare my person class again, boom, put my name property back, I can basically inherit from another object, and that means collect the properties and behaviors of that object and put it into my class.
[00:00:36]
So the syntax to do so would be colon after this. Kinda like when you call a constructor and then you declare the name of the object. The type of the object, I should say, the type of the class. This is a superfluous thing to say, because person will always inherit from object.
[00:00:52]
Object is always in the inheritance hierarchy. So inheritance means that objects can be infinitely inherited. You could have a monkey that inherits from animal, that inherits from organism, that ultimately inherits from object, for instance. This is superfluous to say. But this gets really important when you're starting to talk about kind of advanced ways to model real world things in your application.
[00:01:17]
Let's take this example for instance. We're gonna move on to the employees. And spoiler alert, we're gonna do a lot of employees in the ASP. There's gonna be a lot of employees classes and use of them in the ASP NET Core course. So I can have what I've defined as my base employee and what I've wanted to do is just say, I want an employee and let's just say that for the sake of argument that I wanted an employee where I just wanted that person to be represented.
[00:01:44]
I don't necessarily intend for this type to be used directly, but I just want to have a base thing, because all employees, they always have a first name and a last name. All payroll systems contain that data. They'll probably contain your social, your date of birth and other properties like that.
[00:01:59]
But you might have additional behaviors that you want to apply to something like an hourly employee. And so hourly employees are treated differently from salary. So you may choose in your system to model it as such. So in this case, I've modeled it such that my hourly employee inherits from my base employee.
[00:02:18]
And the implication of that is that if I create that employee object, let's say, and you notice that I don't have any name or any first name or last name declared anywhere on here, nor do I have this method. And we'll talk about these methods and we haven't gotten to the behaviors of classes really.
[00:02:37]
We'll mention that in a second. So if I want to declare an hourly employee, and his name is hourly employee, and we're gonna name him first name George.
>> Spencer Schneidenbach: Last name, Harold, all right. If I go and I look at this hourly employee, even though this is the type that I've instantiated right here, because I've inherited from base employee, I've inherited all of the properties and all of the behaviors of the base employee class.
[00:03:16]
So I'm able to say, yes, I want my first name. Yes, I want my last name. But in addition, I also want my hourly rate because an hourly employee has an hourly rate associated with them. So I can also choose to add that to here, right? And by the way, the M key is just to denote that this is a decimal.
[00:03:38]
There's special keywords for declaring certain types of numbers, and M is the one for the decimal type. So that's in a nutshell, the first part of inheritance. But the rabbit hole gets deeper and it's very important. So I mentioned that classes cannot only have properties, but they can have behaviors.
[00:03:58]
Up to this point, we've really only looked at classes that had properties. That's what I wanted you to focus on, because that's a lot of times what I'm creating classes that just are a bag of properties and don't have behaviors, but sometimes they have both. So you notice that I have a method here called get employee details.
[00:04:16]
And this get employee details has an implementation that basically says, return first name and last name. So if I call get employee details on this person, so Console.WriteLine, employee details. I use method syntax to call it. It does not take parameters, so it's just an open and close parentheses at the end.
[00:04:38]
Otherwise, the compiler will complain, you don't wanna do that. And then I am going to dotnet run, and you can see that George Herold's name is called. Now because of inheritance, my hourly employee has access to this GetEmployeeDetails. Let's talk about accessibility a little bit. This might be a method that I don't want other things to call, not even derived classes.
[00:05:07]
Hourly employees is considered what's called a derived class from base employee. So accessibility can be used to close this method off even from derived instances of this method. So let's say, protected, first and foremost, protected is a keyword that will basically say the only people that have access to this thing are the class itself and the derived class within the class scope.
[00:05:32]
But anything outside of that scope is not something that it has access to. So you can see that now all of a sudden we're getting a compiler error. However, if we wanted to call GetEmployeeDetails from within our hourly employee class, we absolutely could do that. That's something that we can do because it is derived from base employee.
[00:05:52]
So protected basically says anything in the object hierarchy can have access to this method. However, if I mark it private, the only thing that can access GetEmployeeDetails is the base employee class. First things first, we'll talk about this virtual keyword here in a second. Virtual or abstract methods cannot be private.
[00:06:15]
So that makes sense, which we'll explain in a second. So we would say this. And then you would see that now all of a sudden even our derived class can't access this what's called a private member. And that applies to properties as well as methods. So this is the idea of behaviors in a class in addition to just properties.
[00:06:36]
And it also illustrates kind of the importance and significance of accessibility modifiers. Protected, private and public are the ones that I use 99% of the time. I will use one called internal, which means that it's only accessible within the assembly that you have. So the compiled thing that you have and isn't accessible to other assemblies.
[00:06:58]
A little bit outside the scope, just know that it exists if you ever encounter it.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops