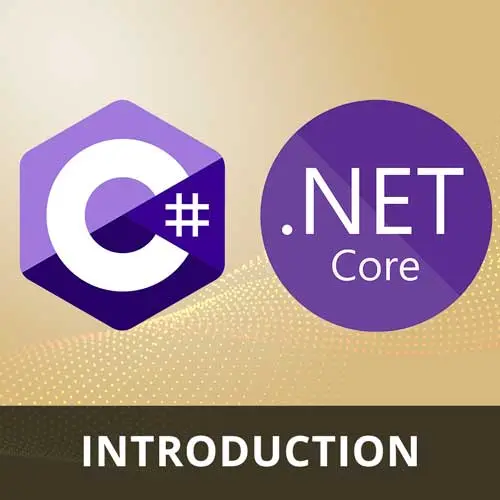
Lesson Description
The "CSharp Built-in Types" Lesson is part of the full, C# and .NET Basics course featured in this preview video. Here's what you'd learn in this lesson:
Spencer discusses various built-in types in C Sharp. He cover types such as int, string, bool, char, byte, long, float, double, decimal, DateTime, arrays, enums, tuples, null, and void. Spencer explains the purpose and usage of each type and provides examples to illustrate their usage. He also answer questions from the audience regarding enums and console output.
Transcript from the "CSharp Built-in Types" Lesson
[00:00:00]
>> Spencer Schneidenbach: Let's talk about some of the built-in types for C-#. So, we've already kind of touched on int, and we've touched on string, we've touched on Boolean. But there are quite a few others, and I kind of wanted to just mention them and run through them fairly quickly just so that we know that they exist.
[00:00:19]
So first of all, all objects, all types inherent from object, even value types, again, we'll get into that a little bit. But basically anything in .net is an object in some ways, including functions, although it's not nearly as function first as a language like JavaScript, but all types inherent from object.
[00:00:40]
So that's the core type in in C-sharp. But we have other we have of course string. We also have single characters or char or char I don't know how you pronounce it, I call it char, and that is denoted with instead of on a double quotes for a string.
[00:01:00]
It's just a single quote. So, if you have a char, and you declare it as just the letter G, you can have the char be set to that value. You cannot have an empty char. You cannot have a char with more than one char character. So that's how you declare them.
[00:01:23]
Not something I declare a super lot of, but important to know for single characters, because there is some important implications to that. I mean, a string is essentially just an array of chars, after all, array of characters. So that's one of the types, one of the core types inside.
[00:01:41]
Boolean represents true or false, a very important part of any language, very important part of any ecosystem to be able to tell if something is true or false. Because we need to be able to operate on certain things based on whether or not it's true or false. I'm sure that the question will come up, is there truthy or falsy in C-sharp?
[00:02:00]
There is not, that is a JavaScript thing, only you're doing if statements and similar things on bools. You're using bools or booleans for that, strictly speaking. So, there are other data types, so like the smallest kind of a number value, or the common value is a byte. A byte is just 8 bit unsigned integer, and an sbyte is 8 bit signed integer, so, pretty typical in most languages.
[00:02:34]
So represent single bytes rarely do I declare them if you're doing any kind of file processing for a specific file format. You're probably declaring bytes to read that file specifically in different places, but most of the time I'm not declaring them. My favorite data type for declaring integers is indeed int, int or long depending on the application.
[00:03:01]
Long just has way more capacity. So in a database system where you're getting a bunch of records, that may be a difference maker in terms of your ability to not hit some kind of upper bound in an auto incrementing integer, which we'll talk about in the next course.
[00:03:18]
But, for the most part, if I'm declaring an integer, I'm just gonna declare it as int. I do that out of force of habit. I do that because most other developers do that as well. Rarely do I see things declared as short unless it's unless it actually is going to be a short.
[00:03:33]
And even then, most people just defer to int.
>> Spencer Schneidenbach: Unsigned integers, shorts, longs are basically it's exactly how they say they don't have a positive or a negative sign in front of the value. It just is a value. So the value for unsigned int would be min value would be 0, and the max value would be double the max value of an integer, because an integer could can be negative as well, negative 2 billion, 147 million, right?
[00:04:08]
That's its range. That's its potential range of values. Most of the time I'm just using int, even for things that I know are never gonna to be negative. Again, it's a force of habit. It's a consistency thing. Float, double, and decimal. So a I'm not gonna get into whole floating point math.
[00:04:29]
I don't think I'd be able to explain it super well, even if I did, but there are those very important data types here which represent numbers that have a well, as they say, a floating decimal. So it could be 31.415, and so we have ways of representing those inside of the language.
[00:04:49]
If I'm representing a floating point value, it's probably going to be a double. But most of the time, just for consistency and for precision, I prefer to use decimal. Decimal does have, it does take aditional memory, but in most cases where I'm using it, I prefer the consistency.
[00:05:05]
I don't really see the memory use. I'm personally a fan of decimals. It just is for consistency reasons, that's mainly what I use. So DateTime, DateTime is a really important value, because dates matter Times matter. So let's talk about that. Let's talk about a few different things that dates have, I'm gonna go ahead and clear out a lot of these things.
[00:05:30]
So first things first, there's a couple of static dates properties that date times have, which in this case are. UTC now, today, and now 99% of the time in a production system, I'm going to be UTC now. So that's going to take the it's going to give me a representation of the current time on the system that the code is executing it.
[00:05:56]
So if I do Console.WriteLine, and I do this, I do open up my terminal, control shift tilde, I do dotnet run. You're gonna see that it does indeed print the current time, which in UTC. So, I said, there's datetime.today and datetime.now, two of which I avoid pretty much at all costs when I'm writing production systems.
[00:06:26]
Something I'd like to note is I mentioned that daytime is immutable. When you're first programming, I remember spending 30 minutes banging my head against a wall, figuring out strings also that they're immutable. And trying to figure out why name two lower did not convert my name to a lower string.
[00:06:52]
>> Spencer Schneidenbach: So if I run this, you'll see that the Spencer variable that I declared here, it didn't get lowered. And that's because these are immutable by default. So you can't change them once they're instantiated, you can reassign the variable that they're part of, which is what I'm doing here, and then we'll get the expected behavior.
[00:07:11]
DateTime has a lot of the same semantics. So if you did DateTime.UtcNow.AddDays, you're not gonna magically add one day to UTC now. You're gonna get a new instance of the of the DateTime object, and you can see that when you dig into the the source code.
[00:07:32]
So you can see that. And I should tell you, I went in order to get into the source code. I think for you it would be I'm not exactly sure what the button would be, but for me it's command. So I command and click. You can see that it gives me underlines and then I can go through.
[00:07:47]
And then I can look at the source code for this. And you can see as we get further in that instance of the date time itself is not altered. The only thing that's altered., That nothing is altered the only thing that's done is a new copy of DateTime is being made.
[00:08:04]
So there are a lot of DateTime methods, so you can get the current year, the current day for that DateTime instance. You can add days, milliseconds, and then get new copies of DateTime. So, very important that you know it, we're not gonna go through every single one of these methods, but it's important that you know that these things are available.
[00:08:26]
DateTime.Parse is a way of taking a string and
>> Spencer Schneidenbach: Declaring a, so if we do DateTime d = DateTime.Parse it's a static method, so that means you don't need an instance to access it. And what it'll do is it'll take in a date, it's fairly forgiving in terms of the format of the date you can specify specific date formatters.
[00:08:53]
Most of the time I let my frameworks serialize and deserialize like a JSON value in a date time. I let Frameworks do that lifting for me. But occasionally it's useful to be able to parse this on its own. If this thing cannot be parsed, it will throw an error, which we will talk about, a little later when we get to talking about exceptions.
[00:09:13]
Let's see, this is my spicy take. This isn't really spicy in terms of most .NET developers. I think most production .NET developers would agree that DateTime.Now is something that we should avoid, and DateTime.UtcNow is the thing that you should be using when you're in a production environment. So with that, moving on to the next one of the collection types, and one of the most important ones is arrays.
[00:09:37]
As I mentioned, that's a collection of data when we looked at the params, kind of method, we saw that there was that open bracket, close bracket, right? And that's just array syntax. So an array is just a an array of a list of values. Arrays cannot be changed.
[00:09:54]
They cannot be resized. They you in order to resize them, you have to redeclare them. That's not like in JavaScript, where an array you can add or remove elements from it, arrays are fixed in size period after they're instantiated. So, if we declare an array of ints, so array of integers, we can use, we can do, this is a fairly new C# feature, but what you can do is have the array syntax here.
[00:10:22]
Note that you cannot declare this as a var. It kind of goes to the vestigial nature of some of C#. It's been around for a long time. It doesn't know the type. It doesn't have a type for this collection and it doesn't want to make an assumption about it.
[00:10:34]
So we have to declare that ahead of time, which is fine. So an array of integers, we have that array. And that is our list of integers. You can also declare an array of integers or an array of anything without any specific values in it. You can give it a number here, and that just basically says that I wanna array of size with three elements in it.
[00:10:59]
Yes Mark.
>> Mark: Can you treat in a string like an array, like you can in Javascript and iterate through the characters?
>> Spencer Schneidenbach: Yes, you can. Yeah, because a string is an array of chars, characters so skipping ahead a little bit in terms of syntax, but char character in hello world.
[00:11:28]
You can absolutely iterate through them and get the I misspelled character, and get each of the characters inside of that string. Good question.
>> Spencer Schneidenbach: Let's see. Most of the time, the one, if you have a multidimensional array, AKA an array that's declared with this. In practice, I have not written code that uses this, that requires multiple or an array with multiple what they call ranks.
[00:11:58]
But you can declare them so that you can have, if you have a coordinate of x's and y's an array that's declared like that, might be a good useful time for that to declare that. But in this case, the one thing that I wanna point out is the length property on the array will tell you how many elements are in the array.
[00:12:16]
It's something that I check a lot, especially if I'm looking to see if an array is empty. I will check it and see if this array is zero, because I wanna be able to operate or do something on that based on that value.
>> Spencer Schneidenbach: Enums, Enums are a set of named constants is the best way to to put them.
[00:12:41]
And so the way that you declare them is with the enum keyword. So these can be declared as a separate type. They're considered a type just like the rest of like classes and things like that. So you can declare a type for or you can declare an enum as a type.
[00:12:59]
You can also an enum has what's called an underlying type and by default the underlying type for all enums is int. And the first value here is equal to zero, the next value here is equal to one, the value here is equal to two. You can also explicitly set them most of the time I am explicitly setting them and I usually start at one and then I go one equals or manager equals one supervisor equals two.
[00:13:25]
But you can set them to different values in case you have maybe there's a mathematical reason you wanna declare an enum where the integers have specific meaning to you. So, you might do that that way. Let's see. I mostly don't assign to enums. I usually just let the compiler do its thing and just use them as kind of just dumb markers unless I'm storing them in a database.
[00:13:50]
And I typically then will definitely start with one and assign them. And the way that you would use them is pretty much like anything else, employee type. We can declare it and then EmployeeType.Manager, you can see that we basically are able to get that type and this will be an enum.
[00:14:07]
So Console.WriteLine there it's when it's written to as a string employeeType will be written as exactly as it looks. So very cool, I use enums a lot as discriminators for different things, we'll have a type of employee or a type of payroll or salary type or things like that.
[00:14:33]
That's relevant for the ASP.NET Core Course. You can also cast them back to their core type. So you can see that if we declare this as int, this will just go back to being an int. So like I said, all enums have an underlying type. In practice, I only use the int type.
[00:14:51]
You can declare them as byte, you can declare them as long, but I only declare them as int, mostly because I'm just using enums as, like I said, dumb markers.
>> Spencer Schneidenbach: So, I believe TypeScript does support string enums. I don't believe, unless they've added it, but C# does not support that.
[00:15:10]
The last thing that we'll talk about is tuples. So, I go into this in way more detail when inside of this documentation, but essentially a tuple is just a set of values. It's a value type, which means that it's stored in fast access memory. And it can be made up of just value types like integer and Boolean, or reference types like instances of classes.
[00:15:37]
And pretty much the way that you, it's basically just a set of values. You can think of it like an array, but it doesn't have to share a value. Tuples are a fairly recent addition to the language. They actually had support for them a while ago, but they weren't very pretty.
[00:15:54]
This one is a lot easier and nicer to use. So if you just declare a tuple without any named Properties, you can access them via Item1, Item2, Item3, kinda not super useful. But not kinda not super useful when you're trying to name things explicitly. And you can give them names, so you could say something like this, for instance.
[00:16:20]
Where you give the tuple meaningful names like this. And then you're able to access them by, very similar to how you would a class variable where you can say, I want personInfo.Age. Or name as and I want to get their name, and so on and so forth.
[00:16:39]
Named tuples are something that I use a ton of, especially if I have something like a method that has a tuple return type. So I will have Int Age and then string Name. If I wanna be able to return multiple values from a method like this
>> Spencer Schneidenbach: Return 30 Alice, I wanna be able to access this such that if I do call this method GetEmployee, boom, I can get age and name.
[00:17:18]
I didn't do it isEmployee, but I can get those things by name. So I use tuples, with tuples I think there's actually a lot of pronunciations for tuple. I don't think anybody [LAUGH] agrees on one. I always say tuple, but sometimes I slip and say tuple, whatever the case, whatever the pronunciation of tuple is.
[00:17:33]
I use them a lot when I want to have multiple return types, return things, values, return from a method. But I don't wanna declare something else like a class to hold those values. We'll talk about that more when we get to classes and types. Let's see, we talked about the use cases, we talked about that.
[00:17:53]
Okay, this is the last thing. So, null is a big thing. There is no, so first of all for those JavaScripters, there's no such thing as undefined in C-sharp, we have null and null is it. And null is basically saying that there is no value for this thing.
[00:18:12]
You cannot do things like set an integer, this integer to null. You can't do that because null is a, the default value for an integer is zero. You cannot declare an integer as a null value. It's somewhere in memory. It's considered what's stored on the stack, which we'll touch on briefly.
[00:18:32]
Null is for things like classes where there's going to be multiple there may be there. It's basically what's it's called a reference type, and we'll talk about that more. We'll talk about the implications of more, you can consider it, it's not really a type, it's more of to say an absence of a type.
[00:18:47]
But it kind of is an important thing to touch on in terms of, hey we need it, this is a thing inside of the language, but we'll touch on it a lot more as we get further in.
>> Spencer Schneidenbach: And then of course void is actually, I know it's a key word to say this method doesn't have a return type.
[00:19:03]
The fun fact is that void actually is a type inside of the CLR, but it's not treated as one inside of the language. And yes, I have tried to instantiate them and no, you cannot do that. I've tried it many different ways [LAUGH] just for fun. But, that is it for types.
[00:19:21]
Can I answer any questions?
>> Mark: Yes.
>> Student 1: Going back to enums, when we did that Console.WriteLine for the employee, type,
>> Spencer Schneidenbach: Yes.
>> Student 1: Is that return value than a string?
>> Spencer Schneidenbach: Yes, that's a good question. So what Console.WriteLine, it's more of a behavior of Console.WriteLine. So you'll see that as you dig in, as you dig into the source code, it's not letting me navigate any further.
[00:19:46]
But essentially, whatever value is passed into this, they call its ToString method. So all, something that's a good thing to note as we get into objects is that objects, there's a a few different things. A few different methods that they all commonly share, one of them is ToString.
[00:20:07]
So you can call ToString on any object and get its string representation. And under the hood, that's what WriteLine or Console.WriteLine is doing is it's calling its ToString method on that enum. And when you call ToString on enum, you get. When you get on the enum not convert, if you don't convert it to its underlying type.
[00:20:25]
You will get if you do employeeType.Manager.ToString(), you will get this word back as a string. Great question. Yes.
>> Student 2: Is there a way to get all the values of the enum?
>> Spencer Schneidenbach: Yes, there is. So that would be done via like. So if you wanted to do that, it would be for each var enum in.
[00:20:48]
We'll just call it E in, and then... There is a System.Enum class, and it has GetValues. And so what you can do is, Getvalues you can pass in the type of that enum. And then be able to iterate through and get all of the values from that and print them or whatever the case may be.
[00:21:08]
Good question. It would look a little bit like this, EmployeeType.
>> Spencer Schneidenbach: And then you could go through and spin through and Console.WriteLine.
>> Spencer Schneidenbach: E, boom.
>> Student 3: And then you run it, and you can see that it will happily chew through and print all of the different enum values to the to the console.
[00:21:36]
>> Spencer Schneidenbach: Good question.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops