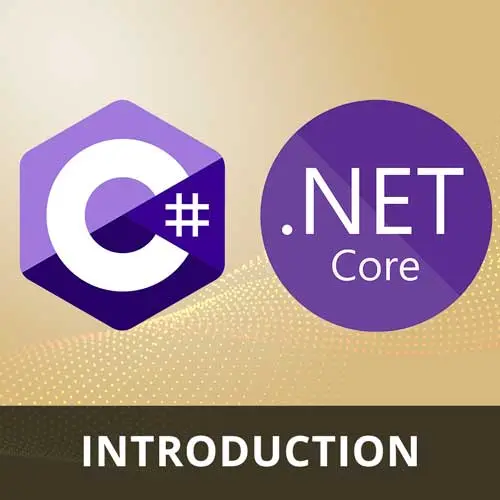
Lesson Description
The "Conversion" Lesson is part of the full, C# and .NET Basics course featured in this preview video. Here's what you'd learn in this lesson:
Spencer explains that C# will automatically convert certain types in limited circumstances. He covers implicit and explicit conversions, as well as the use of parse methods and the convert class for converting between different types.
Transcript from the "Conversion" Lesson
[00:00:00]
>> Spencer Schneidenbach: All right, I wanna touch on conversion, which is another operator. Sometimes C# will do it for you automatically in very limited circumstances. I wanna emphasize that, again, there's no magic, there's no surprises, inasmuch as one would expect from the language. I will start by saying int a = 5.
[00:00:20]
And what will happen is a, it's an integer, right, it doesn't have a decimal place. So, if you assign double b to a, under the hood it will automatically say, okay, well, a can be a double, it's now 5.000, whatever,it would be in a floating point variable. The opposite is not true.
[00:00:44]
Int c, if we tried to assign it to b, we will get a compiler error that says that we cannot implicitly convert double to int. So implicit conversions are fairly limited in C#, you can actually create your own limited conversions. I can't tell you the last time I did, because, again, I don't like magic.
[00:01:05]
Typically I write a function or something else to convert that value for me if I wanted to do that. Explicit conversions, also known as a cast, you could say, hey, I wanna cast this thing to a double. This becomes more important when you're talking about types where at runtime you may get an object variable, but you don't necessarily know the type ahead of time at runtime.
[00:01:33]
So that does happen, that is a thing. Very useful, mostly useful to just know for now, not necessarily useful to apply, we'll see examples of that as we go through. And then, let's see.
>> Spencer Schneidenbach: I mentioned earlier that there is parse methods. And a lot of the core types like int, double, they all have a method that you can use to parse to a string to a value, which is something that you will do quite a bit actually.
[00:02:06]
As you're taking input from text boxes from users, you'll wanna be able to convert those strings. Sometimes the framework does it for you, sometimes it won't if you're writing it a certain different way. So you wanna be able to convert those things into their target type. So if we wanted to say what is my age, we might say int.Parse.
[00:02:28]
And let's pretend it came from a text box, 42 ,and then we say textboxAge, and then we're able to get this age variable. And you can see, as long as it doesn't crash, we know that that parse operation handled successfully. So there's bool.Parse, so you can parse true or false, there's double.Parse.
[00:03:01]
Let's see, I declared it here as an int. So there's pretty much any of the core types, DateTime.Parse, they all have parse methods, static methods that you can use to perform that parse. But there's also one other method for performing the parse that I think is really important, which is the TryParse.
[00:03:23]
So we mentioned earlier about exceptions and knowing when they can happen. You could very easily wrap a try catch around this, and then catch a format exception and then return invalid Console.WriteLine "invalid input", you can do that. It's a lot of code, right? That's a lot of stuff to write to just try one operation, so what you can do instead of this is do what's called a TryParse.
[00:03:57]
Let me show you what that looks like. So, let's declare it as a bool tryParseResult = let's say int.TryParse(textBoxAge). You see, why is it giving me a compiler error? It actually has a second argument, this is a little bit of a new syntax. But what it is essentially saying, there's what's called an "out" that you can return.
[00:04:25]
So this is kind of like a return value from a method, but one that's defined inside of a parameter. This was really important back in the olden days of .NET when out parameters were the best way at the time of returning multiple values from a method. And here's how you would use them back then.
[00:04:45]
You would declare your age here, you would say bool TryParse, and then you would say out age. And the out is necessary, otherwise it will complain at you. Say out age, and then, what you have is a boolean to say, well, is this result successful or not? So if you say, if (!tryParseResult), which is just basically this operator right here, is the negation operator.
[00:05:15]
It says whatever the opposite of this is. So if this is not successful, is what this is saying, then we would print Console.WriteLine("invalid input");, boom.
>> Spencer Schneidenbach: dotnet run, and you can see that because this parsed it successfully, Console.WriteLine("invalid input") didn't run. But as soon as we put anything else in there in a format that it can't interpret, you'll see that it gives invalid input.
[00:05:51]
This is a useful way of testing a result without actually trying to go through the motion of trying and catching exceptions. It's very useful for avoiding that, it helps keep the code concise. I also wanna point out, you don't have to declare your integer down here like this anymore.
[00:06:11]
You can say out var, you can actually declare it up there, and that was introduced I'd say about ten or so years ago, so you can do this all in a single line. So if I have try parse result, and then age, dot, and it will complain to you that, let's see, identifier, explain, Console.WriteLine.
[00:06:35]
>> Spencer Schneidenbach: You'll see that it actually does have a value. And in this case the default value of this is 0, the default value of integer is 0, so it just prints 0. But if try parse result is successful, then the value will be populated. So if we go back to 42 and run it again, you can see that 42 is the value of age variable and it does, indeed, print valid input.
[00:07:03]
So TryParse is on DateTime, int, decimal, lots of different base classes, the base types defined in .NET. So I highly recommend using those, I use those a ton. The last thing that I will mention is the convert class, I would highly advise that you take a look at all of the different things, it's a static class.
[00:07:28]
So that means you just use the methods that are on this class, you can convert things like the string true to the Boolean type. Some of these are very odd in that they will straight up tell you that they will always throw an exception. So if you try to convert a DateTime to a Boolean, it will just always fail.
[00:07:47]
Little vestigial things like that in C# never fail to amuse me. But the convert class is another way as if you know that you wanna be able to convert between types, the convert class is the most best way to do that explicitly. That's how you, for instance, would say, you can't double a,
>> Spencer Schneidenbach: You can't declare an int to a, but you can Convert.ToInt32(a).
[00:08:17]
And what that will do is,you will obviously lose precision, because the 4.2 will get chopped off, but it will explicitly convert it to a. So, again, kind of emphasizing the lack of, quote unquote, magic thing that happens under the hood. I think C# does a great job of protecting you from these things.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops