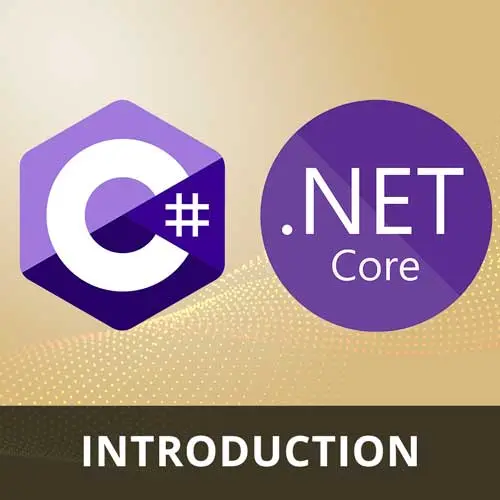
Lesson Description
The "Control Flow" Lesson is part of the full, C# and .NET Basics course featured in this preview video. Here's what you'd learn in this lesson:
Spencer if statements, if-else statements, cascading if statements, switch statements, for loops, while loops, do-while loops, and for-each loops. He also mentions the use of break and continue statements to exit or skip iterations in a loop.
Transcript from the "Control Flow" Lesson
[00:00:00]
>> Spencer Schneidenbach: I do wanna mention control flow, and you've seen a little bit of this, we need to be able to alter how our program executes based on the current state that it's in. So, we've already kind of looked at if statements, whereas if we have this (age >= 18), I'll paste that in here, boom.
[00:00:23]
>> Spencer Schneidenbach: Move that over. One thing Rider does for me is it automatically adjusts my white space. So, if we have our age, and we say that we're greater than equal to 18, it's gonna print you are an adult. There's a couple of different iterations or kind of things of this that are important to call out.
[00:00:43]
So, like in most other languages, you're gonna be able to do an if else. Same thing here with C#, if this condition doesn't get met, it will execute what goes on in the else blocks. So we check there, and we say that if the age is 16 you are a minor.
[00:00:58]
You can also have multiple cascading if statements where you can also check other conditions. You can say, if age is greater than 18, Console.WriteLine("You are an adult."); { else if (age >= 13) ("You are a teenager.");, and so on and so forth. One thing that's syntactically important is that some languages do not require that you have these parenthesis here.
[00:01:19]
C#, they are required, so I wanted to make sure to call that out. Switch statements are another one if you have a long list of values and you're really only checking one of those particular values, and you're checking it multiple different ways to see if it equals certain things.
[00:01:38]
A switch statement is a great way to do that. So let's just copy this code into here, and you can see that based on our day of the week, boom, boom, there we go.
>> Spencer Schneidenbach: Format that nicely, there we go, based on the day of the week it's going to print a different string.
[00:01:57]
Some things to note about the switch statement, the switch always begins with switch at the top and then the variable that you're checking. And then you will go down inside of this curly brace that indicates a block of code, and it indicates the block inside of the switch statement.
[00:02:10]
And you'll see, for case 1, it will write Monday, first day of the week is Monday in certain cultures, right? Case 2 is Tuesday, and so on, and you see that because of our day is 3, it gets evaluated to Wednesday. One of those vestigial parts of C# is you'll notice this break statement.
[00:02:33]
We don't have the break statement there, the C# compiler can complain at you and say, no, you have to break this switch statement before you're able to exit it. So it's something that is required. There's also a default case, you can also have a default case. So if you're checking this for a value that doesn't fall within the 1 to 7 range, it will just say invalid day.
[00:02:56]
So if it's a value that it can't be checked,
>> Spencer Schneidenbach: It'll write Invalid day, so it'll go down to this default case, which is pretty useful. It's important to note that switch statements in this particular way only operate on value types. So you can have switch statements that operate on reference types in different ways, that is pattern matching, which is a relatively new feature in C#, and we'll cover that just a little bit later.
[00:03:23]
You can see, by the way, so, it's recently that switch expressions have been introduced to the language in the last few years, and that is a way that you can more concisely write a switch statement. I personally think that the syntax is a little bit cleaner, but I don't really see that it has this super big advantage, other than it does save lines of code.
[00:03:46]
It doesn't have that break statement, but this is functionally equivalent to the switch statement that you have above. You have the variable that operates on the switch, and then the same kind of logic executes based on the value of the day. So there's no real difference or real surprise here, this that you're seeing here is functionally equivalent.
[00:04:07]
I do wanna talk about loops, of course, one of the most important control structures. This is a classic for loop that you see in lots of different languages. It's made up of three statements inside the parenthesis, the declaration statement where you declare the variable, the statement where you check to see if the loop should continue to execute, this returns boolean.
[00:04:33]
And then a statement usually made to increment the number or change the condition of this to see if the loop should keep executing. So, as you might expect,
>> Spencer Schneidenbach: If I copy and paste this code in here, this loop will execute 5 times, it will basically say start at 0, and while i < 5, write Console.WriteLine.
[00:04:59]
And after every time the loop runs just increment that variable, so you can see that it writes out number 0 to 4. Pretty standard, like I said this is the classic loop, it is not a loop that I write a ton of very much anymore. Most of the time, if I wanna iterate, I'm usually iterating over a collection, and in that case, I'm using foreach.
[00:05:19]
So, while loop is a basically a way to continue to run a loop while a condition is being met. I write a surprising amount of these. Oftentimes I will write them like this, while (true), and I'll do some kind of logic and then eventually exit the loop. One of the ways you can exit any loop at any time is you can write a break statement, and that will exit the loop immediately.
[00:05:45]
So what I'll do is I'll just say basically do this process as many times as you need to until I get a result that I expect. As you can imagine, this is also a fairly dangerous way to work on a loop, so a lot of testing needs to go into any time that you write a while (true) loop.
[00:06:00]
But you don't have to just have while (true), you can also have some condition. So if you have int i = 0 and then you say while (i != 5), Console.WriteLine(i), and we do i++.
>> Spencer Schneidenbach: You will see that it will print numbers 1 through 5. And then as soon as this condition is false, it will exit the loop and it will stop.
[00:06:29]
Now, of course, we could do while (true) and then really spin this thing out of control without a break statement that would inevitably crash the program. [LAUGH] So, as I mentioned, most of the time when I'm using while loops it's in some kind of form or fashion like this.
[00:06:48]
It's a little bit advanced, because you do have to be careful with that. The do-while loop is a condition where everything that's going on in the do will execute at least once. I do not see these often, I do not ever use them, not because they're not useful.
[00:07:04]
Occasionally, once maybe a year, I will do a do-while. But this is basically where you wanna say, I want the do statement to execute at least one time and then continue to execute based on the value of a condition. So, fairly useful to know about, not super important to write about.
[00:07:25]
Foreach is a loop that is pretty much the loop that I use for anything. Anytime I'm using operating on some type of collection, I'm probably doing a foreach loop. So let me declare real quick an array of values. And you saw me give an example of this earlier, but I basically can say value in arr, and then it will go through each of the elements of this array and I can do some operation on them, so Console.Writeline(value);.
[00:07:58]
I don't need to know anything about this array, like its length, I don't need to track some kind of i in order to track what my place is, the language and the runtime do that for me. So this is probably the most common loop that in professional development you will use, because you will iterate over a collection of things and do something with them.
[00:08:18]
Pretty cool, I mentioned break. Break is a statement that you can use to exit a loop at any time that goes for foreach as well. So if I do foreach break;, boom, and I hit play and run this, it's not gonna return anything, because I just broke this loop and just exited before it could even run.
[00:08:38]
The continue statement is used to shortcut your way out of a loop, in case, for some reason, you need to exit the or wanna continue the loop, but you don't want some other logic to run. So let's print Console.WriteLine(i); and you'll see that the loop does not stop executing.
[00:09:02]
It continues, just as the statement says, but it does skip 5, which is exactly what we expect, it stops here. You can think of it as kind of like a return statement for a loop in that it just shortcuts and then it exits out of the loop very quickly.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops