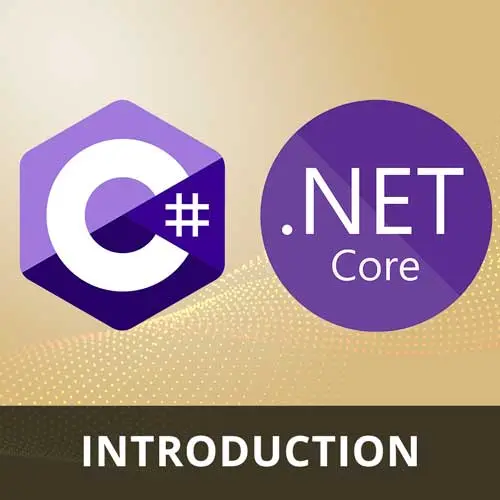
Lesson Description
The "Classes" Lesson is part of the full, C# and .NET Basics course featured in this preview video. Here's what you'd learn in this lesson:
Spencer introduces the concept of classes in object-oriented programming. He demonstrates how to declare fields and properties in a class and explains the difference between them. He also shows how to control the accessibility of properties and how to create computed properties.
Transcript from the "Classes" Lesson
[00:00:00]
>> Spencer Schneidenbach: All right, now let's move on to classes. This is the most exciting part, I think. And there's a lot to cover. So let's just get right into it. We've already kind of alluded to what a class is. A class is, just like this is defined here. It's a basically a thing, and a definition for something that can exist, right?
[00:00:23]
This thing is a person class and it can, and a person can have a name and it can have an age, right? So they're a fundamental building block of object-oriented programming and what you can do is you can describe things in the real world. You can describe their structure, and you can describe their behaviors.
[00:00:44]
This is a object-oriented pillar of abstraction, right? These things have meaning in our systems, even though a Spencer record may exist in a database and probably exists in lots of different databases. That thing has meaning, even though it's not me. That's what classes are meant to represent, as things in the real world that have meaning.
[00:01:04]
So a class is pretty much defined same way as in a very similar way as a method, but they're obviously very different in that there's an accessibility modifier followed by the class keywords called the class keyword. And then the name of the class, I should mention that classes are Pascal cased in C Sharp.
[00:01:27]
And I think that's pretty well true in JavaScript, as well as that, there tend to be Pascal case, especially in the TypeScript world. So classes can contain all kinds of things. I wanna look at fields real quick. So this is what's referred to as a property. And we'll get to this syntax.
[00:01:47]
It looks a little silly but we'll get to it here in a second. Let's instead declare this person as using a what's called a field, and a field is just an object on this class. It belongs to this class. So fields are very common to use, but not necessarily common to use directly on classes that kind of represent a data structure.
[00:02:17]
And you'll see that when we talk about properties. But you can see that using this, which is called dot notation, I can take in a person that has a specific name, and I can access that name property, Console.WriteLine, and I can use dot notation to get into that and access that name.
[00:02:46]
And you can see, if we hover over it, it says it's a field on the person class, and it's called name, and it's type of string.
>> Spencer Schneidenbach: And if we run that, you can see that we can access that just fine. Wait a second, what did I do here?
[00:03:00]
Person console.WriteLine.
>> Spencer Schneidenbach: Let's try dotnet run. Okay, here we go, old name. Which is what I have the value of my person class set to, or the name on my person class is set to, is old value. So properties are what I would consider to be a more important and the main focus of when you're talking about classes, we should be talking about properties.
[00:03:31]
Properties are an abstraction over fields, and what that means is that that they property is meant to control access to a field, because you may not want to reveal a field to other classes. And here's basically back in the olden days of C sharp, when you declared a class and declared a property, you would have to declare a field with, typically, fields that are private, AKA accessible only by this person class.
[00:04:01]
You can't do person_name, it will complain that is inaccessible due to its protection level. So you can't access it because it's private to this person class. But we can expose it out using the name property, and we would say, get return _name, which would return this value, or we would set the value of name of the _name field to this value.
[00:04:27]
This is the syntax that used to be, this is what you would use to declare properties in C sharp. Luckily the syntax is a lot friendlier and my main way of declaring them is like this, this syntax automatically creates a field under the hood as well as the property.
[00:04:49]
And I'd say it's a lot nicer than having to declare the field separately and then explicitly set up your getters and setters, as what these are referred to as,
>> Spencer Schneidenbach: This is the more concise way, and by far the superior, 99% of the time when I'm declaring properties this is how they look.
[00:05:09]
They're public. They're always have a type declared, and then they always have a name and then a getter and setter, which we can modify accessibility for. And I'll show you what that looks like here in a second. So one of the main use cases for properties is being able to set and get fields, but only by choice.
[00:05:29]
Let me demonstrate. So let's take this example of a person class that has a name property that does not have a setter, as you can see if we try to set it, the compiler will complain and say this is read-only. We can actually set this property, but we can only do that in the context of what's referred to as a constructor, which we will get to very shortly.
[00:05:52]
The important thing to know is that a property can appropriately hide or specifically disallow getting or even setting it. The getters and setters can also have accessibility modifiers, so we can say private set. And you can see that if you hover over this, that the compiler still throws an error, but it throws a slightly different one.
[00:06:16]
Says, hey, I can't get to this property because it can only be set inside of the context of this class, in this case, you could set it if you declared a A method, I would never do this in practice. I would just open the setter up as necessary.
[00:06:30]
This is look looking like very Java like now, but you could do Name = name and then you'd be able to set the value of this person object's name. Because we still have the setter open, it's just not exposed outside of the context of the person class. Hopefully, you're getting a sense of kind of how accessibility modifiers operate.
[00:06:54]
Public and private are the two ones that we've kind of mostly gone through now. Deleting this will instantly convert it to a public setter by default because it will follow this accessibility modifier here. Consequently, if you take that off, it's considered a private property and you will not be able to access it here as well.
[00:07:15]
Again, most of the time, I'm using classes to define real objects in the real world and I'm usually keeping the getters and them setters open on certain things, for objects that represent real life things. Lastly, properties can also be get only, but also just have some kind of computed value.
[00:07:33]
They're called computed properties, and they're super useful. So assuming that we have a class that has a FirstName and a LastName. We can also have a FullName property on that class that essentially combines the FirstName and the LastName. Or you could even do it like this. You could say even just LastName +, so you can concatenate strings with the plus operator and then say FirstName.
[00:08:02]
So you can do that as well. It's called a computed property. This syntax, this equals and greater than looks a little funky. As we get into Lambda functions, you'll see why that syntax is existed the way it has. It's pronounced goes into, a little spoiler alert. But that's essentially how you can create a read only property that allows you to get data off of that, but you can combine it in useful ways that your developers will appreciate.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops