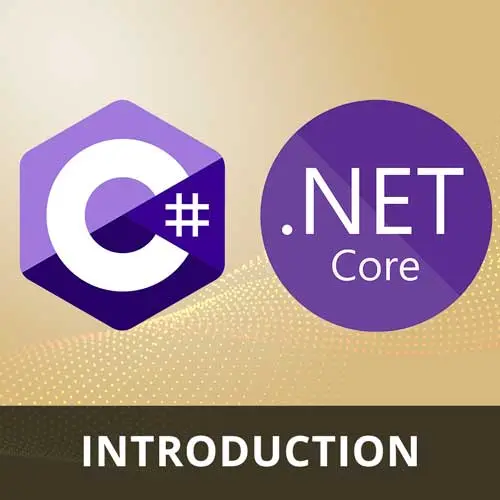
Lesson Description
The "Arrays vs. Collections" Lesson is part of the full, C# and .NET Basics course featured in this preview video. Here's what you'd learn in this lesson:
Spencer explains that arrays cannot be resized or have elements added or removed, and that if more space is needed, a new array must be created. He also introduces the IEnumerable interface, a generic collection type that allows for iterating over collections and performing actions on each item.
Transcript from the "Arrays vs. Collections" Lesson
[00:00:00]
>> Spencer Schneidenbach: Okay, I wanna talk about arrays again. Because they are important, at least in the context of this. We're talking about collections now. So when you declare a set of arrays, you can declare an array of integers in this case, we're declaring it with five elements and we've just set all of those elements to the empty value.
[00:00:19]
We could set those values individually. We can get the value for a value that we haven't set, which just results in a zero because that's the default value of int. And then we can try to get something outside the bounds of that array, which would throw an index out of range exception.
[00:00:37]
So arrays, as I mentioned earlier are fixed. They're fixed length, you don't add and remove from them like you do in JavaScript. That just isn't a thing. So once you declare them, they cannot be changed, they cannot be resized, you have to create a new array if you need more space, and you can't remove or add elements.
[00:00:58]
So early on in .NET Days, there were collection types that kind of dealt with that thing. I'm not gonna go over these, because, in practice, these aren't used anymore unless you're dealing with really old code. But you could essentially have a list where it essentially you can add or remove things from this list.
[00:01:16]
Problem is that these weren't constrained for any particular type. So an array list could exist where you added a one, excuse me, an integer. An array list could exist where you could add an integer and then the next line, add a string and there was no type safety.
[00:01:29]
There's nothing behind that to prevent that from being from occurring. So I want to talk about the generic collection type called IEnumerable <T>. IEnumerable<T> is one of the most important constructs inside of the language, and it's very simple. It essentially says that it is a collection of things.
[00:01:49]
An array of objects implements IEnumerable <T>. An array of ints is an IEnumerable<int>, okay? And it basically says, hey, we can do things with this collection. We can iterate over them, and that includes doing things like foreach. Remember, I mentioned the foreach loop, and I mentioned that we use it a ton in the real world, at least, I certainly do.
[00:02:11]
So we can spin through each of these items and then write them to the console. It's also known IEnumerables in especially functional Programming languages are also referred to as sequences, but I just refer to them in this context as IEnumerables. Something that you should know about IEnumerables is that as they are being created, they are not always accessed right away.
[00:02:32]
So they may not actually run until you actually start spinning through and requesting items from them. This will become really important. It's called lazy evaluation. And I just want you to put a pin in that term and think about it as we talk about LINQ (Language Integrated Query) a little bit later.
[00:02:48]
You can define what's called an iterator method. This is a rather advanced thing to do because there are programming, there are performance, and there are kind of semantic implications to doing this. But what you can do is you can define a method as returning IEnumerable and then use the yield keyword to actually start yielding back or returning back items inside that IEnumerable.
[00:03:13]
This is really powerful and really cool. A little bit outside the scope of this course, I would recommend that as you learn more about LINQ, it'll start to click more. And there's documentation here that really talks about what's going on with yield under the hood, because it's actually fairly complex.
[00:03:31]
So it's a good thing to know, but not really important for right now. Put a pin in it just a little bit later.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops