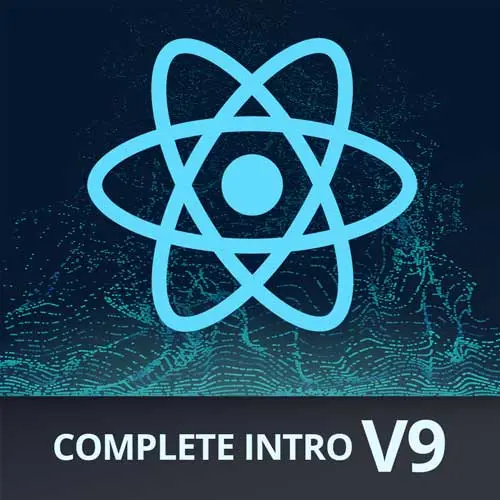
Lesson Description
The "useState Hook" Lesson is part of the full, Complete Intro to React, v9 course featured in this preview video. Here's what you'd learn in this lesson:
Brian introduces the useState hook and creates hooks for the pizza type and size. Hooks provide mutable properties along with setters for changing the values. The setters are called in the onChange event handlers for the select element and the radio buttons.
Transcript from the "useState Hook" Lesson
[00:00:00]
>> Brian Holt: But let's talk a little bit about what we've done here. We have an order form, this will eventually will look nicer once we have all the pieces put together.
>> Brian Holt: I want you to try and select a different type of pizza.
>> Brian Holt: Doesn't work, right?
>> Brian Holt: It broke the browser, which is actually kinda hard to do, right?
[00:00:31]
The browser works really well at creating these types of forms, and whatever we did with React made it not work, which seems kind of counterproductive, right? Same thing with the pizza size, click on one of these other buttons. No matter what, you're always going to be selecting small or large, and that's annoying, right?
[00:00:50]
We expect the radio buttons to work so that you can select small, medium, or large. Well, reason with me of why this is happening.
>> Brian Holt: All right, you have [COUGH] pepperoni here, right? Which is the value here, so that's why it's selecting that one. Now I click Hawaiian, right?
[00:01:14]
If I click Hawaiian here, what happens? React says, hey, an event happened, the user did something, it's time for us to re-render this component, right? So it goes through and it re-renders this component, says pizza type pepperoni, right? And so it sets the value to be pizza type pepperoni.
[00:01:35]
So even though the user is clicking on something else, it's immediately setting it back to where it was, because this isn't dynamic, right? This is just assigned and then it just stays that way. So we have to do some sort of assigning pizza type to be something different or else it's just gonna continually be that.
[00:01:57]
>> Brian Holt: This is just a roundabout way of me explaining and showing to you that two-way data binding is not free in React. There are some other frameworks. Famously, Angular 1 was a big two-way data binding framework. And that's just not true anymore, we found that that's very fun to write and very bad to maintain because it becomes very black magic of where did this variable come from?
[00:02:22]
>> Brian Holt: Part of the magic of React is that it is verbose. I'm not gonna argue with you that it is not. It could be shorter or more terse or something like that. It's a little less fun to write, and it's a lot more fun to maintain. And as I've gone through my career, I have found that that's actually worth its weight in gold, right?
[00:02:41]
I don't wanna optimize for the couple of hours I'm gonna spend writing something. I wanna optimize for the hundreds of hours I'm gonna spend maintaining something.
>> Brian Holt: Okay, so that's my spiel of why I think this is okay, despite the fact that it is a little annoying.
>> Brian Holt: Interesting, if you go try something like solid js, which is very similar to React in many ways.
[00:03:06]
They found a way to kind of make it work with something called signals. I think signals are in pre-act as well, no, I haven't written pre-act in a long time. But anyway, suffice to say other framework, different approach to this, but React takes a very straightforward, very, I wanna call it JavaScripty.
[00:03:23]
They're just kind of working with JavaScript to make it work the way that JavaScript normally works, as opposed to having black magic around the the framework to make it work differently. So let's, let's go make this work with state then, right? We're gonna use something called a hook.
[00:03:42]
Everyone do this thing called input, use state from React. Anything that you see use in React, the alarm should go off in your head, it's like this is a hook, okay? So instead of these two, I'm gonna say const pizza type, set pizza type.
>> Brian Holt: Equals use state, and then you give it the default first value, pepperoni.
[00:04:20]
Same thing with pizza size, const. SetPizzaSize equals useState and whatever you want the default to be. We had it as medium, we'll just leave it as medium, but you could change it to large if you're trying to drive up your margins or something like that.
>> Brian Holt: This is also a course on capitalism, I don't know if I told you that, [LAUGH].
[00:04:48]
All right, so now we have this use state thing, but this now makes pizza type able to be set to be something different. It makes this React components stateful, but we still have to call this set pizza type somewhere so that we can change it. So the way that we're gonna do it is we're gonna go on our select here.
[00:05:12]
By the way, these are just highlighted because they're not being used anywhere but. We'll do that in just a second. All right, so I'm just gonna put some space here so you can see what I'm writing. We're gonna do something here called onChange. And the only thing we're going to do is we're going to say e, which is like a normal break.
[00:05:40]
Browser event, right? You could call this event just for so many years of doing this, I just always call it e. So that's really up to you,
>> Brian Holt: And we're just gonna give this this anonymous function, let's say set pizza type to be e.target.value.
>> Brian Holt: So this is saying whenever a change event happens on select call this function.
[00:06:12]
This function takes in an event and it calls set pizza type with the target.value, the target is gonna be one of these options, right? Because that's what's actually gonna be the Well, I think the target's actually the select, not thinking about it, but the value will change, right?
[00:06:29]
So the value will be whatever the option is. So this value will be whatever their user has set, and we'll call that with set pizza type, which will then end up changing the pizza type up here. Which kicks off a re-render, and now it's gonna be correctly rendering the same thing.
[00:06:45]
So if we set this size is still not gonna work because we haven't done that yet. But let's go try this one. We should be able to set this to something else. Congratulations, we unbroke it, right? All right so this is called a hook. Some rules around fight club, we don't talk about fight club.
[00:07:12]
Some rules around hooks, you do talk about hooks, you can talk about hooks, that's fine.
>> Brian Holt: They can never be inside of conditionals. So never, ever do something like this. If something right, then use state. This is a big no no, because the one thing about hooks is that they depend on being called in the same order every single time.
[00:07:38]
So, if I did a conditional there, then it would sometimes give them to me out of order. So really what would happen is sometimes like medium would show up in pizza type, which is not what you want. So, in other words, conditional or hooks never go inside of for loops, while loops,, do loops.
[00:08:00]
They never go inside of conditionals, they're just always top level. Even if you don't use them, that's fine. Just never put them in conditionals. You never have to. So you just, sometimes you ignore a hook and that's fine, but you still have to call it in the same order every single time.
[00:08:16]
There's linting rules that will help you with that. As well, so why are they called hooks? In my like head canon of why this is, I don't I've never asked anyone this. This is just what occurs to me, is there's a hook that gets called in the same order every single time.
[00:08:35]
So it's like a hook being picked up, right? And they get picked up in the same order every single time in these render functions, right?
>> Brian Holt: Yep, hooks rely on strict ordering, we talked about that. Yeah some of you might be thinking like, this seems like a weird API, right?
[00:08:56]
It is kind of a weird API, if you think about it. I'm just so used to to seeing it so frequently that, again, a frog that got boiled, right? But it's just returning to you an array of two things, the actual thing, and then the setter for the actual thing, right?
[00:09:12]
That's just all that is, right? So you could definitely say, like const, pizza hook,
>> Brian Holt: =useState
>> Brian Holt: Right, and then you would say const pizzaType = pizzaHook[0]. And then set pizzaType.
>> Brian Holt: Right, this one line is the equivalent of these three lines. But we're all very lazy and we all write it this way.
[00:09:52]
So useState is. By far the most common one that you will use, but I will show you a couple other hooks. But just know that use state is by far the most common one. Yeah. Someone online is just asking if you could console log just to show kind of the state changing.
[00:10:14]
Sure.
>> Brian Holt: Type, pizza size,
>> Brian Holt: All right, let's just trash this.
>> Brian Holt: All right, so, you can see every time that a user event is happening here, it's actually doing a re-render. It's interesting that it is smart enough to know that events happening in other parts of the Dom are not being observed, and so it's not catching those events.
[00:10:59]
Reactors gotten a lot smarter over the years. They used to would have triggered a re-render, and it doesn't anymore. So let's go make it work with pizza size as well.
>> Brian Holt: So with, pizza size there's a couple of different ways to do this. I'll show you the lazy way that the linter will complain about.
[00:11:24]
>> Brian Holt: Event bubbling still works in React as well. So in theory, you can actually do it this way
>> Brian Holt: Equals, same thing, E, setPizzaSize to be E.target.value,
>> Brian Holt: And then this will start working Working, right? Because we have one event listener on the parent element, right? And so it's listening for just the one time.
[00:12:04]
React doesn't like this because it views as inaccessible, which generally you don't want change handlers on like divs because screen readers and all that kind of stuff can't access. divs, they access inputs, right? I think that in this particular case, it wouldn't matter because it's still bubbling the event no matter what.
[00:12:24]
And so you can have a change event on a input and it'll still work. But let's just appease the linting gods. And we're going to put this on all the individual inputs. It's more explicit as well, so we're just gonna put this three different times in all of these.
[00:12:48]
>> Brian Holt: Now you might say, well do I need E.target.value? Or should I do S here like this.
>> Brian Holt: Tomato, tomato, same thing.
>> Brian Holt: Because the value is coming from here, right?
>> Brian Holt: But in both cases, it works.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops