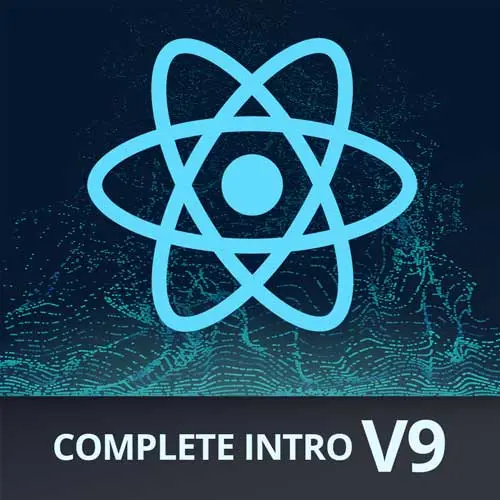
Lesson Description
The "TanStack Query" Lesson is part of the full, Complete Intro to React, v9 course featured in this preview video. Here's what you'd learn in this lesson:
Brian introduces TanStack Query, an asynchronous state management library with declarative, always-up-to-date auto-managed queries and mutations. TanStack Query is installed and configured in the project, and the developer tools are also added.
Transcript from the "TanStack Query" Lesson
[00:00:00]
>> Brian Holt: So let's talk about Tanstack query. This is the artist formerly known as React query. They call it now a Tanstack query because now it works across several libraries, not just React. There's an angular query, there's view query, there's a couple of them. But I would say far and above the most popular one is Tanstack query.
[00:00:23]
So we're gonna make a past orders page, and this page is going to pull in all of the old orders that we can kind of navigate through them. What's interesting about this data is it's highly, highly, highly cacheable, right? Your old orders aren't really changing from page load to page load.
[00:00:43]
You might be getting new ones, right? But the old ones are gonna keep staying there, and particularly, if you click the details, right? The details of an old order aren't changing minute to minute, so very highly cacheable. That's kinda the nice thing about Tanstack query, is that it makes caching API requests just so simple.
[00:01:06]
Imagine right now with everything that's shown with use effect, how would you cache a response? You could, right? I imagine most of you would make some sort of object and that object would live from you may be another external module and you would set a key And then you say if I'm requesting this, then don't request it pull it from cache.
[00:01:24]
But no one wants to write that logic, right? Then you have to write the expiry logic. You have to write like hydration logic if you wanna preload it. There's a bunch of stuff like that and you don't have to because ten stack query kind of does it for you.
[00:01:37]
It's almost to the point that I almost. To never have to use effect anymore. I just use Tanstack query for almost all the things I was using use effect for. So, with React, whenever I start a brand new project, it's NPM install, React, React-DARM and Tanstack query, almost all the time, right?
[00:02:01]
Everything else, I don't always need routing, I don't always need the other tools, but I almost always end up using tenstack query, just because it's better and easier to use. And use effect, it's just as you can see, can have some pretty strange bugs. You just kind of sidestep that by using tenstack query.
[00:02:17]
So I'm a fan, as you can tell. So Let us go make our past orders page. It's gonna be fun, if you have a warped sense of fun, like I do. All right, I'm gonna run my server again. NPM run dev, now inside of routes, I'm gonna make a new file.
[00:02:38]
We're gonna call this past.lazy.jsx. And it's gonna create this really nice little stub for us thank you Tanstack.
>> Brian Holt: And that all looks good.
>> Brian Holt: So before we get too far here, let's go ahead and install all of our things that we need. So I'm just gonna grab this directly out of the docs.
[00:03:04]
We're gonna be using Tanstack five 5.59.13 today.
>> Brian Holt: Again, this library doesn't really change too too much between versions. There's some experimental features that I'm sure that they'll end up adding later, but we're not using any of the experimental Features today. Actually, that's not true, towards the end we will, it'll be fun just in another terminal here.
[00:03:26]
I'm gonna run MPM install at Tantack/React-query. Here we're gonna install MPM install TanStack React query. Add 5.59.13, so ahead and run Install there. Then we're gonna grab one out of here as well. This is just a bunch of them. It has some really nice dev tools and it has some nice linting rules that we're gonna bring in as well.
[00:03:53]
So npm, install dash D, cuz these are dev dependencies. Tantack react query dev tools, ojhave the warick. Is like the router dev tools. I'll say that I use the query ones more than I use the router ones, so these ones are actually more useful in my opinion. And then we're gonna install the plugin as well for it for ESLint specifically.
[00:04:16]
And again 5.59 13 and 5.59.7 are the versions I'm using.
>> Brian Holt: Awesome, let's go modify our ESLint config here, put that there and then. So we're going to import at the top, import, plug in. The query from @ tan stack/eslint-plugin-query.
>> Brian Holt: And under the JSX runtime one here we're just gonna say ...pluginquery.config,
>> Brian Holt: Flat/recommended.
[00:05:14]
>> Brian Holt: I think I've complained about this style of config seven times in this workshop, but hear me out one more time. This is just nonsensical-looking. Some of them are spread, some of them are not. Frankly, I spent a lot of time getting it right before this course, right?
[00:05:38]
Anyway, that was my problem, but not yours. But your future problem. So, maybe we can commiserate. All right, so that should work. I mean, it's always good to just give her an all NPM run link just to make sure that say like, something broke that's cool.
>> Brian Holt: Flat, recommended all right, so let's make sure that I got this right here.
[00:06:01]
PluginQuery.configs with an s.
>> Brian Holt: Right there. So I had config, it's configs, let's try that again. There we go, okay, good.
>> Brian Holt: Get my dev server running again, all righty.
>> Brian Holt: So let's go ahead and add our dev tools, might be fun, could be cute. So we're going to root.jsx.
[00:06:43]
And here at the top, we're going to,
>> Brian Holt: I don`t know, wherever you want to, under here, import,
>> Brian Holt: ReactQueryDevtools like that, for. From at Tanstack, react query dev tools,
>> Brian Holt: okay, and then I just put it like here. It doesn't really matter, but we'll just put it like right there.
[00:07:14]
React query dev tools, like that.
>> Brian Holt: Some of these dev tools things are just popping up now. Cuz I think there's a Vercel one and a Next.js one, and I think React Toolkit might have one. So you can have like seven sets of DevTools in your app. It's going to end up being like those, like old school, like like web 2.0 browsers that have like 17 ads that all pop up, but it's going to be just DevTools everywhere.
[00:07:51]
Can't decide if that's like utopian or dystopian, and I think the answer is both.
>> Brian Holt: Okay, so now we have dev tools in there. I think if you just look at your app now, yeah, so it's mad that we still haven't put the actual React query library in yet.
[00:08:08]
It's like, I'm looking for something to hook onto, and I can't find it yet. So that's expected. So app.jsx, we're going to have to import query client and query client provider from that Tanstacked React query here.
>> Brian Holt: So those are the two things I'm importing here. We have the create router here and then we have to create underneath that a const query client equals new query client.
[00:08:51]
There's some configurations you can pass this I don't remember what they are necessarily off the top of my head.
>> Brian Holt: You can pre fill the cache, which is kind of cool, it's covering it. So let's say you have a bunch of requests that you know that the user is likely to make, if in our example.
[00:09:15]
Let's say we could pre fill this with like the last ten orders, just knowing that that's the most likely orders that people are going to look at, right? You could pass that in in query cache, right? And so you can precache these things, which is kinda cool. The reason why I really like that is now all of our API requests get to look normal.
[00:09:34]
We don't have to say, all right, check the cache first and then make the API request. It's like, nope, just make the call tanstack queries just be like, if I have it, I'll give it to you already, and if I don't have it, I'll go get it. Mutation cache is much the same.
[00:09:47]
Mutations are like post requests, right, where you're like posting something to the API. You can cache that it's a bit more weird. And then the default options here, queries, mutations, hydrate, dehydrate, there's a bunch of options around doing stuff like that. Those were just the TypeScript types. If that was confusing to anyone, you can just command, click on things, and it'll take you to the TypeScript types.
[00:10:09]
I do that all the time, just to, it's like, what does this do? Why does it do it this way? Sometimes the the types can help.
>> Brian Holt: Okay, so we have that. Now, we have to give it to the app with the QueryClientProvider. Client = queryClient and we'll just do it like that.
[00:10:39]
>> Brian Holt: There we go, so now if you look at our page, it's loading again, and you can see we have this nice little palm tree down in the corner. It's very tropical, and you click on that, we don't we're not using it yet. So our dev tools are vacant, but stuff will start showing up here, which is kind of cool you.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops