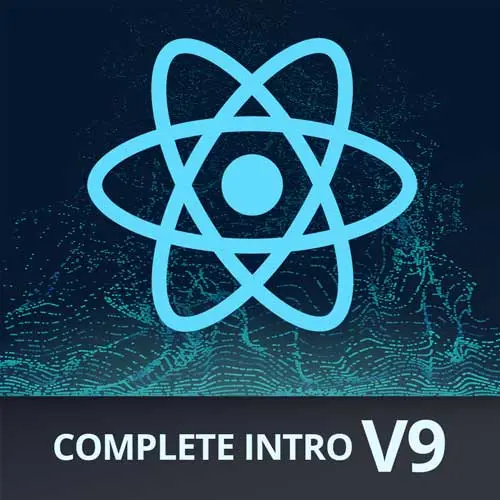
Lesson Description
The "Strict Mode & Dev Tools" Lesson is part of the full, Complete Intro to React, v9 course featured in this preview video. Here's what you'd learn in this lesson:
Brian installs the React Dev Tools and uses them to inspect the application. The React Dev Tools provide React-specific details like the component rendering tree, state properties, and what effects were called. A profiler is included in the Dev Tools as well.
Transcript from the "Strict Mode & Dev Tools" Lesson
[00:00:00]
>> Brian Holt: So this is just kind of a brief overview on some helper things that you can do with React just to kind of make it easier to work with. So one of them is, we're using vite. So vite will take care of NODE_ENV for you automatically. But NODE_ENV, if you're not familiar with it, it's just basically telling the tools that you're working with is, am I in development mode, or am I in production mode?
[00:00:20]
And usually it's assumed that you're in production mode, and then you only set it to development mode when you're actually doing dev work. This used to be a thing that you had to set it explicitly, and that's why I still mention it here. So, if you're doing Webpack, you might still have to set it explicitly of I'm in dev mode right now.
[00:00:36]
This is important to React because React has a lot of helpers and better error messages and all that kind of stuff when you're in dev mode. But the actual executable of React is a lot slower and it's four times bigger. Or at least that's what it was, I haven't checked recently how big it is, but it was quite big.
[00:00:56]
So if you're following along with me, if you're using Next, if you're using Remix, they all set NODE_ENV for you automatically. If you're using something like Webpack directly, you might have to set it yourself.
>> Brian Holt: One of the reasons I bring this up is that make sure this gets set to production, when you actually do go out to production, that becomes important.
[00:01:17]
Famously Slack shipped, React, and dev mode for a very, very long time and they actually saw a massive increase in performance in Slack. Because all they had to do was switch NODE_ENV to production, right? Which is a very dumb reason to waste a lot of people's time and resources, right?
[00:01:34]
So that's one strict mode, let's go do that, right now.
>> Brian Holt: So strict mode is a kind of react mode you can set things in. So we're just gonna go to app.jsx,
>> Brian Holt: And we're gonna say at the top import strict mode. From React, and we're just gonna wrap our entire app in its strict mode.
[00:02:13]
>> Brian Holt: So what is strict mode? It's just like an extra set of checks that React will do things for you normally. But in strict mode it'll say hey, you're executing in strict mode, I'm not going to let you do this. Usually what it is, is if you're using things that are about to be removed from React, it'll give you a big warning.
[00:02:34]
It's like, hey, this has been warned that this is gonna be removed from a future version of React. Am not going to let you use this because you're in strict mode. So they do that so that people know in advance that stuff's gonna be removed from React. So that's what strict mode is.
[00:02:50]
Yeah, continue to double renders your components, just and make sure that all the effects are run twice, right? It can catch subtle bugs, of sometimes you have effects that are doing something one time, and working differently another time, that's kind of what they're supposed to catch. If you're asking me, how many times has that caught something for me?
[00:03:07]
The answer is not really, cuz once you're in the mindset of how effects and state is supposed to work, it's pretty rare that you would get outside of that. Last thing here, we're going to install the DevTools. So I have a link down here for you, this should take you to the React docs, and they have dev tools available for Chrome, Firefox, and Edge.
[00:03:32]
They used to have it for Safari, but I guess no one uses Safari, so they took it away. No one uses Firefox, but I'm happy they still maintain that one. So yeah, let me go show you Firefox. So you should just be able to click in here and say add, I've already installed it so mine's gonna stay there, it's the same thing with Chrome.
[00:03:55]
And then what's cool about this,
>> Brian Holt: Let me make my devtools a little bit smaller. You should see down here at the end, components and profiler.
>> Brian Holt: So you get two little things that you get added by the React dev tools. And so let's just go look at Components.
[00:04:19]
You can see here I have an app component, Order Pizza, this is kind of the expected order of components that we have inside of our app here. If I click on Order, I can also see all the hooks that happen, which is pretty cool, right? I can see everything coming in from, these are the pizza types, right?
[00:04:39]
You can see I have a pepperoni, medium. Here's something kind of fun you can do, you can actually edit this directly. So if I hit this to I think it's something like that, I break it, that's cool.
>> Brian Holt: There we go. All right, so notice, this is barbecue chicken.
[00:05:00]
I can actually edit this directly to be pepperoni. And notice when I hit Enter, this is gonna become pepperoni, right?
>> Brian Holt: Sometimes that can be useful, right? I can edit this to be small. It can be useful for debugging just to see, if I change this to be this, what happens?
[00:05:24]
>> Brian Holt: Yeah again, here, you can see all the props that come into this one. It does let you edit props, that used to not be true, how cool is that?
>> Brian Holt: So that's that. I'll show you one other trick that I find pretty useful. All right, so, let's say I wanna investigate this radio buttons, I can click Inspect here, right?
[00:05:54]
So I click Inspect. Obviously that takes me into the inspector, it doesn't take me into the React dev tools, but if I just go over to the React dev tools.
>> Brian Holt: Usually it'll take you directly to that that component, but it's probably because it's in the
>> Brian Holt: Larger component.
[00:06:15]
Yeah, this time it did, okay so that time it took me over to the pizza, right? Right now we only have three components. By the end of this course, we'll probably have 50-ish, just because it'll introduce things like the router that have a lot of layers to it.
[00:06:32]
>> Brian Holt: That can be super useful for finding a component deeply buried in a tree. The last thing that I'll show you is that we have that and now I can hit $ + R.
>> Brian Holt: This is the component that I have selected in the React components, right? So you can see this is actually the pepperoni pizza, it's the last thing that I selected in the component tree here.
[00:07:03]
Which is more of a party trick than it is useful to be honest with you. I don't find myself wanting to programmatically interact with it very much, but it is there, which is pretty cool. This is totally unrelated to React, but I don't know if you click on this and you hit $ + 0.
[00:07:21]
You'll get the last thing you selected in the inspector, I find myself doing that quite a bit more.
>> Brian Holt: So I introduce those now to just so that for the rest of the course, if you have any questions or concerns, you can reference them, kind of as we go.
[00:07:40]
There is the profiler as well, we're gonna talk less about that, but let's say if I just start recording and do a bunch of stuff here. Sorry, don't do that right now.
>> Brian Holt: All right so blah blah and I click Stop.
>> Brian Holt: Our flame graph isn't super interesting right now, neither is the ranked view.
[00:08:10]
But this is gonna show you kind of the performance profile with the flame graph. If you have a big React app, and you wanna see what is going slow and what's broken down, this is designed to help you with that, specific to React.
>> Brian Holt: These are kinda cool, I hadn't seen these before.
[00:08:29]
So the way that React works is it has commits, right? So they're basically packets of things that have changed. You can see what caused the update, how long it took, what the priority was. Cuz you can have deferred and immediate updates. There's all sorts of fun stuff you can do.
[00:08:45]
We're not talking about performance so much in this particular course. We might touch on that more in intermediate or maybe in a different React performance course.
>> Brian Holt: One thing, just kind of as a general note with React, it's generally fast enough. And when I say that, you kind of get to write React and be fairly naive in terms of not worrying about performance, not worrying about commits or updates or anything like that.
[00:09:11]
My advice to you is just continue writing React that way for the most part. Only introduce the performance enhancements when you have a problem. So stay to differently solve problems you have, don't try to anticipate problems that you might have, because you usually are wrong about what the problems you're about to have.
[00:09:30]
And while the performance enhancements for React work, they work great, they make the code harder to read and harder to debug. And that's I say don't do the performance enhancements until you need them, because it becomes harder to work with your code.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops