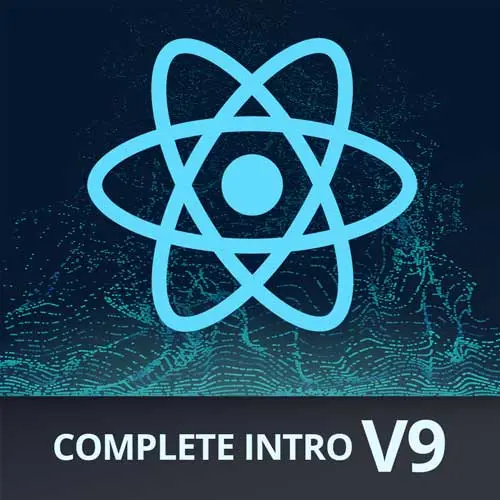
Lesson Description
The "Rendering the Modal" Lesson is part of the full, Complete Intro to React, v9 course featured in this preview video. Here's what you'd learn in this lesson:
Brian adds the JSX to render the modal. A Link component is wrapped around the past orders order_id. When the ID is clicked, the Modal is opened, and the order details are displayed.
Transcript from the "Rendering the Modal" Lesson
[00:00:00]
>> Brian Holt: What's cool about this modal is we really can put it anywhere, I'm gonna put it right here at the end. And this is where I was saying is, I probably would normally split this out into a separate file. But I don't want to today, so we're not going to.
[00:00:14]
So focused order, if we have a focused order we wanna show our modal, right? And if we don't have a focused modal, then what do we wanna render? Nothing, null. You can render null, that's okay. Null just means don't render anything.
>> Speaker 2: Is that better than doing just fragments?
[00:00:38]
>> Brian Holt: Just something like-
>> Speaker 2: Or, yeah, that's what I've usually.
>> Brian Holt: My intuition is it doesn't really matter. I like null cuz you're expressing is I literally want nothing to be here, yeah.
>> Speaker 2: Yes, kinda what I [INAUDIBLE].
>> Brian Holt: But again, we're splitting errors here.
>> Brian Holt: All right, so modal, and then now we can put whatever we want in here.
[00:01:00]
So let's put our model. So we're gonna say Order # and then it's gonna be focusedOrder.
>> Brian Holt: Okay, and then we do have to make sure that it's loaded, right? Because the user will select their order, and it'll render the modal, but we still need to wait until the stuff is actually loaded from the API.
[00:01:32]
So we're gonna say that when it's not loading past order,
>> Brian Holt: Then do this otherwise, again, null, or actually I think I have a loading there. Yeah, I do all right. So this is gonna be, what do I have? Is it a p? Yeah, it is a p, <p> Loading ...</p>, like that.
[00:02:02]
Otherwise, we're gonna do another one of our favorite things, tables, again. <Table> <thead>, you were not sick of hearing me say that, <tr>, <td>, and it's Image. Name, let's just copy and paste a bunch of these. I need Size, Quantity, Price, Total. So this is Size, this is Quantity, this is Price, and this is Total, okay?
[00:02:40]
Under the thead, we are into the <tbody>.
>> Brian Holt: Okay, and then inside of here we're gonna do our map of all the data that we're getting back from the past order data, {pastOrderData.orderItems.map}. And each one of these is gonna be a pizza that we're getting back from the order API.
[00:03:10]
And then inside of that you are gonna have a <tr key=,
>> Brian Holt: I have some longer key here, all right, sounds good. Again, it just has to be something unique to the particular thing that we're looking at. So I did (`${pizza.pizzaTypeId}`}, and this is why.
>> Brian Holt: If you ordered seven of those Napolitano snake pizzas, it will collapse that down to the same quantity.
[00:03:55]
So you're guaranteed that the combination of a pizza type Id and the pizza size will be unique, that's why I did it this way. Again, we're not gonna do any reordering here, so it's actually kind of not necessary for us to be so guarded with our key. But my recommendation for you is, let's say you become the new Domino's and you built this pizza system for it.
[00:04:20]
Maybe later you do need to add the ability to filter and reorder and stuff like that. And it's better to just build these things now, especially since it's usually not very hard to. All right, so for this <td> we're gonna do an >img_src={pizza.image}, and the alt is {pizza.name}.
[00:04:46]
>> Brian Holt: And then we're gonna have a <td> of name, right, {pizza.name}. And again, I'm just gonna be lazy and copy and paste this a bunch of times, Size, Quantity, Price, Total. Size,
>> Brian Holt: Quantity, and then we have our price converter, right? So we're gonna use our priceConverter that we just built, {pizza.price}.
[00:05:18]
And {priceConverter(pizza.total)}
>> Brian Holt: Okay,
>> Brian Holt: That'll be good for our rows, and drew to body, the table, the loading. And we need some ability for us to be able to close. So before this, just I need a button right there. That's a close, how are we gonna close our modal?
[00:05:56]
Maybe a better way of asking the question is, how do we unrender the modal? What is modal rendering based on? FocusedOrder, right? So what happens if we set focusedOrder to be null, or undefined, or zero?
>> Brian Holt: It'll unrender it, right? It just won't be there anymore. So if we say onClick=, and we could just say straight up setFocusedOrder, like that.
[00:06:29]
So we have to go create inside of our API directory, we're gonna create another file here called getPastOrder. Not confusing, definitely not right for us, typo errors, nothing to see here, move along. Export default async function getPastOrder() {.
>> Brian Holt: And this takes in an order number. And we're gonna say const response = await fetch (`/api/past-order/${order}`);, just like that.
[00:07:20]
Const data = await response.json(); and return data. Calls the API looking for a past order with that, await response.json(); and return the data. All right, so we have passed here, this is loading. So we still have no way to open the page, right? We haven't added the ability to click on these rows yet, so we still have to go do that.
[00:07:52]
Go into your PastOrder, or your past.lazy.jsx, and go to where we're rendering our buttons here. So for the order_id we're gonna make that clickable, yep. So here, on line 50 here, we're just gonna put another HTML element here, it's gonna be a button onClick. We're going to just set the order or the focus order.
[00:08:29]
SetFocusedOrder to be (order.order_id).
>> Brian Holt: I don't think I need to do much more than that, nope. Except, we'll just put the order_id inside of it. So all I did was this, I surrounded this order_id with this button here.
>> Brian Holt: Now, again, remember, someone's gonna click this button, it's gonna invoke this function, it's gonna set the FocusedOrder to be whatever the order_id was here.
[00:09:06]
What's that gonna do? It's then gonna open the modal because this is just looking for whenever a focused order exists, right?
>> Brian Holt: Pretty slick, right?
>> Brian Holt: All right, so now we have nice looking buttons here. If I click one of these I get a nice little looking modal.
[00:09:36]
>> Brian Holt: All right, and then, again, notice, when I click these, these ones you'll see just the briefest flash. And let's this do our silly thing and intentionally delay it.
>> Brian Holt: I'll wait for ten seconds.
>> Brian Holt: Okay, so that's gonna make this wait for ten seconds,
>> Brian Holt: Whenever we get there, okay?
[00:10:15]
We're gonna click this one, and then now see it's gonna be sit here and load for a while.
>> Brian Holt: And whenever that finishes, we should end up seeing our nice looking pages here. But again, now if I click it again, because it's pulling from cache, it's not hitting that function, it's gonna be instant.
[00:10:42]
>> Brian Holt: And again, if I hit this one, it's gonna make us wait, right, cuz that one's not in cache right now. So again, I was kind of talking about you can actually preload the cache. So if you're doing some sort of server side rendering, or something like that, or some sort of optimistic loading.
[00:10:57]
What you could do is you could go load the first ten orders, just because you assume people are gonna be mostly looking at the first ten orders, and stick those in cache. And so you would have to do that ten second wait every single time. Or, fun fact, you can just go and delete the await here, that instant performance for free.
[00:11:15]
[LAUGH] This was obviously the most dense part of what we just did. I would say this is one of the more advanced React concepts, or maybe more advanced client-side React concepts. Once you get on the server side, some weird stuff starts to pan out.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops