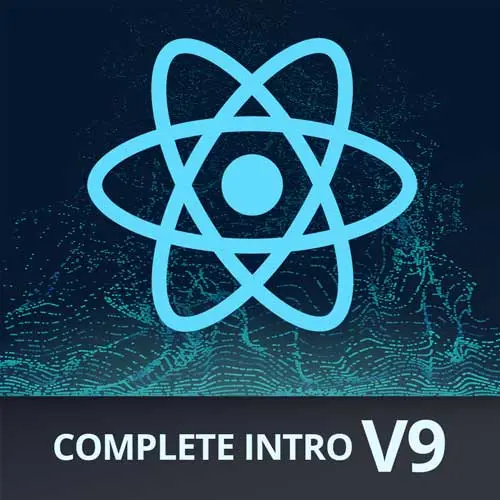
Lesson Description
The "React without a Build Step" Lesson is part of the full, Complete Intro to React, v9 course featured in this preview video. Here's what you'd learn in this lesson:
Brian codes a basic React component. The component is rendered to the page, replacing the "not rendered" text in the <div> tag. The React and ReactDOM libraries are imported using <script> tags, so no build script is required to run the application.
Transcript from the "React without a Build Step" Lesson
[00:00:00]
>> Brian Holt: Let's hop in and start writing some React so that we can eventually make Padre Gino's. So you can see here, I'm in my complete intro to React V9 project. The way I'm gonna do this is I'm just gonna make a new directory in here, and I'm just gonna start working out of that directory.
[00:00:17]
I think that's just gonna be the easiest for me. Yeah, let's do that. We'll just called Padre Gino's, cuz that's what we're gonna make, okay? And then I'm gonna open this in my VS Code editor. So Padre Gino's, empty directory. We're gonna make a index.html.
>> Brian Holt: Okay, and we're gonna start building React with no build step.
[00:00:51]
So we're gonna write React the way that I don't know of anyone that actually writes React without a build step, but I wanna show you that it is actually indeed possible. So one of my favorite cheat codes in VS Code is that there's Emmet built in. So I can just say, html:5, and then I can just hit Tab, and it just generates a valid HTML document for me.
[00:01:16]
So I'm gonna do that. And I'm gonna call this Padre Gino's.
>> Brian Holt: Okay, and then I'm gonna have a div that's gonna be root. And I like to put something in here because this will get replaced. And so if you see not rendered on the page, you know something went wrong.
[00:01:41]
>> Brian Holt: And then we're gonna put a couple of script tags on here. So we're gonna do script, source. If I were you, I would just grab these directly from my page here cuz otherwise, it's really annoying to write by hand.
>> Brian Holt: Unpkg was actually created by one of the React router authors, and it's basically just a CDN full of every NPM package.
[00:02:20]
It's really nice. You can do things like this where you can just load things directly off the CDN and get started with something off of NPM with no build step, which is kinda fun. So unpkg.com, we're getting from react18.3.1. Umd stands for universal module definition, and we're grabbing react and react-dom.
[00:02:44]
Just a quick note on why there's two packages. React is the universal interface for React, so things like React Native, React 3D, React 360, all of those use the same React package. And then the actual rendering layer underneath it that's specific to the browser is called React-DOM. So if you're ever working in a browser, you'll always just have these two packages.
[00:03:10]
If you're working in React Native, you'll have a different React Native toolkit, but it'll still use the same React package. Okay, I'm gonna save that, and then I'm gonna open my terminal here. And we can do a fun little trick here that I'll do, npx serve, just like that.
[00:03:32]
>> Brian Holt: It'll ask you, is it okay if I install this package, and you can say yes. And this will actually just start serving it directly on port 3000 here, and you can see I'm getting this page up on localhost:3000. There's a trillion ways to run a local host server.
[00:03:51]
This is just a very fast one using Node. But you could do php-s, there's a Python one, you can run NGINX via Docker. You could literally just open this directly with Cmd+O, and open it in the browser. So we have this, it's opening on our page, it's using Serve.
[00:04:10]
Serve is made by Vercel, in case you were curious. You get some nice little server logs here, it's kinda fun. We have our two script tags here. Unpkg from React and React-DOM. And the last one, we're gonna put one more script tag on here, and this is just gonna go to ./source/app.js.
[00:04:36]
>> Brian Holt: As you might imagine, we're gonna create a directory here. We're gonna call it Source, and we're gonna create a file in there, and it's gonna be called App.js. So inside of App.js, we're going to create our very first React component. So we're gonna say, const app =, I'm just gonna do an arrow function here.
[00:04:59]
If you've never seen this fat arrow before, this is my ligature combining, which is the font, right, combining in equal signs and an angle bracket. That's what a ligature is. I left a note in my setup part of the course, if you wanna know how to do that.
[00:05:18]
>> Brian Holt: Okay, and then I'm gonna return React, nope, not that, createElement.
>> Brian Holt: Okay, and then we're gonna put in a div, an empty object. You also could put null in here, both of those are fine. And then another React.createElement of an h1 empty object, and whatever you wanna call it.
[00:05:50]
I'm gonna call mine Padre Gino's.
>> Brian Holt: Okay, then we're gonna go down here and say const container. We're gonna grab this, where did I put it? This one, root, right? So we're gonna say, const container = document.
>> Brian Holt: GetElementById, root. Const root equals ReactDOM.createRoot[container]. And then we're gonna say, root.renderReact.createElementApp.
[00:06:52]
>> Brian Holt: That's it, that is your very first React app right there. What do we expect to happen? This is my favorite question before, and I do this all the time when I'm writing code. Before I Click Save and run and see what happens, I always ask myself, what do I expect to happen before I run over there?
[00:07:09]
Well, I have an app thing. You might call it a component. I don't know, if you're feeling spicy, you could call it a component. And it's a type of component, and it returns whatever the result of this createElement is. I'm gonna guess that React.createElement returns an element. I don't know where I got that, but it's probably a good bet there.
[00:07:35]
And there's gonna be a div. This thing, it's like attributes, so it's probably not gonna have an ID, or a class, or a for, or anything like that on it. And then inside of it, there's gonna be an H1 that's gonna say Padre Gino's inside of it. This is going to be rendered inside of the root ID div on the page.
[00:07:58]
All right, let's go see if we're right.
>> Brian Holt: Pretty cool, right? Let's see if we were actually correct. We have a div. Let's see if I can make these dev tools a little bit bigger.
>> Brian Holt: There's ID root there, right? That's the one that we first put on the HTML doc, and then there's a div inside of that.
[00:08:24]
There's an h1 inside of that, and there's a Padre Gino's inside of that. Kinda what we expected, right? All right, you're done, let's call it a day. [LAUGH] No, I'm just kidding, there's a few more things to React than just this. But I'm also gonna say, this is kinda most of React, which is kinda cool.
[00:08:49]
The principal core iteration loop is not terribly complicated with React, which is one of my favorite parts about it.
>> Brian Holt: So React is all about making components, right? We made this app component that every time that you call it, you're gonna get a div, an h1 inside of it, and a text in there that says Padre Gino's.
[00:09:13]
And right now, you can see that we're using createElement again, the exact same one. Here we gave it a string, right, and that gave us the actual literal div tag on the page. So we have the div, we have the h1, and then we have this component. I like to compare this to a stamp, right?
[00:09:31]
So we made this app stamp, and now we can go stamp it as many times as we want, right, cuz it's a reusable component. You could imagine you have a pizza one cuz that's what we're literally about to create here in just a minute. And if you have five different types of pizza, you can stamp it out five different times, but you're creating your little stamp here.
[00:09:51]
And then what createElement does, the only thing that it does is it just stamps the stamp once, right? So I'm calling the React.createElement here with app, and I'm just saying, hey, inside of route, go stamp app once, right? And you get an instance of the class or an instance of the component.
[00:10:09]
So, element instance, you can kind of associate those two words together, component class, you can kind of associate those two words together. If you're an object-oriented programmer, that'll make a lot of sense to you, if you're not, you're just saying words now and making me feel weird, right?
[00:10:26]
I get it, anyway, app reusable component, that's the part that you really wanna take away from this.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops