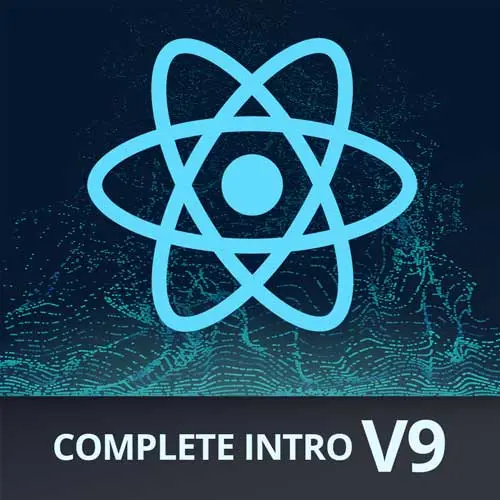
Lesson Description
The "Prettier" Lesson is part of the full, Complete Intro to React, v9 course featured in this preview video. Here's what you'd learn in this lesson:
Brian introduces Prettier, a formatting tool that fixes spacing and stylistic issues in source code. The default Prettier configuration is used, and it is installed as a VS Code extension and a command-line tool. Both are installed because the extension will work with VS Code and fix errors on save. The command-line tool is great for working on teams when developers have different coding environments.
Transcript from the "Prettier" Lesson
[00:00:00]
>> Brian Holt: I take a little bit of a break from writing React, and we're going to talk about tools, which seem to bother everyone but me. I love tools, that's why I've worked in Dev Tools for a very long time. I've shown you enough vanilla JavaScript React for you to know exactly what's happening underneath the hood.
[00:00:17]
From here, we're going to move into JSX, which was a huge barrier to adoption for a lot of people, especially early in the life of React. Where they're like, hey we don't really want to write this compiled to JavaScript language, it feels weird. But we'll get there in just a second, for now we're gonna set up all the tools so that we can do that.
[00:00:37]
So the first thing is we're gonna use npm, I'm gonna use npm for the life of this course, feel free to use Yarn, previous versions of this course have used Yarn before. pnpm, you can see I have a call out to that one as well, I think that's getting more and more popular, but it's kind of hard to go wrong with just straight up npm.
[00:00:58]
If you're not familiar with npm, it does not not stand for node package manager, but it kind of stands for node package manager that's what they say. They say it doesn't stand for that, and then they just finish the sentence and don't say anything else, it's kind of annoying.
[00:01:12]
Anyway, it is the package manager for node js, and it allows you to install these things directly off of the MPM registry, which is now owned by GitHub. Which is in turn by owned by Microsoft. It allows you to do things like npm run or npm install react and things like that.
[00:01:29]
So in our project, let's go do the first thing here, you can go ahead and stop serve, we won't be using that anymore, that's control c if you're not familiar with the Linux. All right, so I'm gonna do npm init dash y, the dash y just says I don't wanna answer any of your dumb questions, just generate a file for me.
[00:01:53]
But if you wanna answer the questions, just leave off the dash y and you'll see that you'll get out of here a packaged JSON. Here, and this allows you to start saving things to your project, the reason why this is useful to us is that we can say, npm install react.
[00:02:09]
It'll go and solve React for us, and it'll store the version of React that we're using in the project. So that when I share this with you later, you can go install it and you'll get the same version of React or a compatible version of React, that I'm using.
[00:02:22]
But I don't have to save React in my repo, so we don't have to ever have a trillion copies of React floating out on GitHub right, we get one copy of React. And if React has a critical vulnerability, stuff like that happens, they're able to patch it so that next time you go to install it, you'll get the patch version not the insecure version.
[00:02:44]
>> Brian Holt: Okay hopefully that worked for you if not you'll probably have to go to the node.js page. And install node however you choose to do that but again and make sure you end up on node 20.6 or above please.
>> Brian Holt: Feel free to use pnpm, it does relatively the same thing, I'd be very confident that it would work just as well Yarn, less so these days I haven't used Yarn in a long time.
[00:03:17]
>> Brian Holt: You might have noticed when I've been writing code here, that if I do something like this, let's close this come on. So if I do like this and I put a bunch of space and then whenever I hit save, Slash [INAUDIBLE] Thank you it just fixes all my spacing for me automatically, I cannot be bothered to handle spacing anymore like that.
[00:03:44]
That process in my brain that thinks about code formatting has just been shut down and at this point it's been shut down for so long, it's attribute. So I literally cannot fathom how to write well formatted code anymore because prettier just doesn't for me automatically every single time.
[00:04:00]
So, Prettier is a code formatter, it takes in unformatted code and it spits out formatted code, it's my favorite tool in JavaScript ever. It's literally changed the way that I write code and blessed be to the Prettier gods. So, let's go ahead and start this project for Prettier, all you have to do is create a file called .prettierrc, and then I put the very controversial empty object as my config.
[00:04:37]
Which is just saying use all the defaults.
>> Brian Holt: My least favorite thing is a bike shedding about code formatting, I hate it. I have been in so many meetings over the years of like, we should be using tabs versus spaces or commas at the end or automated semicolon insertion or not, all those different things.
[00:05:03]
And my message to you is I can't fathom any caring for it, it's my least favorite thing to talk about. So that's why I put this empty object here is whatever someone else chooses for me, I don't care as long as it gets formatted in a uniform way, I don't care.
[00:05:19]
So that's what that is. I have a VS Code extension here so if you're following along in VS Code I'm going to suggest you install the Prettier code formatter. There's several so make sure you get the one that's officially from Prettier and then go into one of your JS files.
[00:05:36]
You can hit it's either shift command p on a Mac or ctrl shift p on Linux and Windows and then choose Format Document. If this is your first time doing it, it's gonna say, hey you need to choose a formatter and just choose Prettier and then that'll set that up so that formats your documents.
[00:06:00]
And then if you hit command comma or control comma, and then you wanna do format on save and just make sure that that one's checked.
>> Brian Holt: Then you're ready to roll. And then you'll get exactly the same thing, so you can just mess up formatting, make it terrible.
[00:06:21]
Let's de-indent everything because that just, it makes everyone ill to look at that code and you just save it and it just fixes itself. I know I want everyone to use VS Code, but I also used to work with the Primeagen, so [LAUGH] not everyone's gonna use VS Code, turns out, right?
[00:06:40]
So you need to set up all of your projects to work with anyone's tools, not just your own tool. So let's go ahead and set up Prettier to work with everybody, npm install --save-dev or if you're lazy like me, npm i -D is the exact same thing. And we're gonna put Prettier.
[00:07:08]
>> Brian Holt: And you can see here we have a devDependency right, so this only needs to be installed in development environments, not for protection environments. That's what the devDependency means. And then, under scripts, we're going to do this, I'm going to set format and you're going to do prettier dash--write, and we're just going to say, do everything in the source directory.
[00:07:49]
>> Brian Holt: And then you need to do js, jsx, ts, tsx, whatever file formats you wanted to look at. That's probably a pretty good list, we're not going to have any TypeScript in this, so you could leave the TypeScript stuff out, but js, jsx, css, you could put HTML in here as well.
[00:08:11]
Preview doesn't work with everything, but they're actually expanding it to a lot of other languages now, make sure you need a comma there at the end.
>> Brian Holt: And now we should be able to run npm run format, that's what the scripts are for. It's basically, please remember these bash commands for me so later so I don't have to remember how to write this.
[00:08:37]
You can just say npm run format and it'll run that for you, and I needed a closing quote here. Make sure you have the quotes here, the backslash is just an escape character, right? The reason why you want the quotes here is that you don't want your shell to do the file expansion.
[00:08:52]
You want the actual, you want that passed in literally as a string to Prettier so that it can go do all the finding of the correct files.
>> Brian Holt: Now, mine's already running right, because every time I save the file, it's running, but you can see so it says unchanged here, but if I had something to change there, it would say.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops