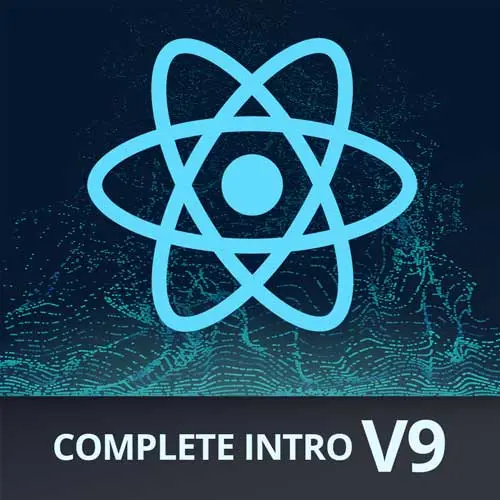
Lesson Description
The "Handling User Input" Lesson is part of the full, Complete Intro to React, v9 course featured in this preview video. Here's what you'd learn in this lesson:
Brian implements the onSubmit event for the add-to-cart form. The preventDefault method stops the default form behavior, and the selected pizza is added to the cart state. A Cart component is created to display all the pizzas in the cart.
Transcript from the "Handling User Input" Lesson
[00:00:00]
>> Brian Holt: So we wanna have a cart. Right now, we have a pizza type. We have a pepperoni that's kind of like displays to one that you have selected there. Now we wanna have a cart over here where base people can click add to cart, and it's just hard refresh the page right?
[00:00:18]
Go to Order.jsx. We're going to import cart from./cart, which we are going to build momentarily. One more hook here, const cart setCart = useState other empty array and then on the form here. So form on submit, we want to write a function here with the event e.preventDefault. That's probably not gonna be surprising to anyone that's written form code before.
[00:01:01]
It prevents it from being submitted, right? And then we're just gonna setCart and we're gonna say ---cart. And then we're just gonna add the pizza that we, they have selected. So it's selectedpizza, size Is pizzaSize and price. Yeah, it's bad right now because I don't have a cart component.
[00:01:27]
That's not real yet, that's fine. So event, we're used to that, preventDefault. This is another spread operator. So the other thing we could have done is like cart.concart, right? And then put in that, or cart.push would have worked as well. This is just another way of doing it called the spread operator.
[00:01:48]
I say like, hey, spread the entire cart before this and then add one more object after that. That's all that does. Someone might ask me, hey, why don't you just put an onClick here instead of a onSubmit up there? You can, but let me tell you why I think this is better.
[00:02:10]
What happens if you have a text input and someone hits Enter? What happens? If you have just an onClick handler, nothing happens. And people expect when they're on a form and they hit Enter, that it submits the form, right? So that's why onSubmit is better, because it handles both of those cases.
[00:02:30]
Generally speaking, it's just more accessible that like screen readers and tools like that are very good at handling form elements. And so if you just kind of hook into the way browsers normally work, you get kind of all the accessibility for free.
>> Brian Holt: Okay, and then just inside the last closing div here, we're gonna put one more thing.
[00:02:51]
We're gonna say if it's loading, then we're gonna say h2 loading. And if it's not loading, then we're gonna say cart is assigned cart cart cart, cart cart cart. The cart component, the property that we're gonna pass into it is the cart. And then we need to go build the cart after this.
[00:03:22]
>> Brian Holt: Okay, so, please, if you will, make a Cart.jsx. Let's make another price formatter. We might be running into the territory of wet write thrice. So I might end up making a function, if I was, this was my project I might end up making a function that like handled that, or it could be a hook.
[00:03:49]
It doesn't have to be a hook, but it could be a hook. Because it's just literally just one function. The only thing is like, imagine if you were doing internationalization and you did want to switch between currencies, you'd have to go change this in every single place, whereas if it was one place, you could do it.
[00:04:06]
But no one does that. Doesn't seem like a real thing people do. Just kidding, people do that all the time. That was my job at Netflix. Currency and then style. What am I doing style is currency and then currency is USD.
>> Brian Holt: Okay, export default function cart. Now I could put props here.
[00:04:43]
But you can also destructure, because that can be fun sometimes, so we're gonna put cart and checkout in here. Let total =0. We need to tally up how much stuff is in our cart, so let's do that first, for let i = 0. i is less than cart.length, i++.
[00:05:14]
And then we're just gonna say const current = cart i and total + = current.pizza.sizes, current.size.
>> Brian Holt: Okay,
>> Brian Holt: Someone might say, hey Brian, why don't you use a reduce here? And to them I say, go back to Haskell. A former Brian in a former life would have written this as a reduce, but I realized way later that I was writing reduces because I thought they were fun and not because I thought they were clear.
[00:06:03]
And so when I run into a lot of maps and reduces and stuff like that, I tend to just like, no, this is probably just easier as a for loop. It makes it more readable to not just you, but to everyone around you. So that is why I do not write this as a reduce.
[00:06:17]
There's something extremely satisfying like writing like those long chains. But usually that satisfaction is smugness and not actually because it's good code. Anyway, div className=cart. The h2 we're gonna put here is cart and then we're gonna do a ul because it's an unordered list. And then we're gonna say cart.map.
[00:06:49]
And then we're gonna do item index.
>> Brian Holt: And we're gonna do the forbidden thing that I told you not to do, where we're gonna use index as the key, why? Because none of the things in our cart are guaranteed unique, right? You can have three medium pepperoni pizzas, in which case, what would be, there is no thing there, right?
[00:07:13]
So this is a specific case where using the index is the key, is what you're supposed to do
>> Brian Holt: Okay, span className = size, item.size, and we have three of these, I'm gonna make that one type, so this has to be .pizza.name, I guess. Okay, that's fine. And this has to be price.
[00:07:53]
So you have size, size, pizza.name, and item.price, cool?
>> Brian Holt: Then we're gonna do pTotal is, and we're gonna use our international format total close that. And then we're gonna write another button, onClick. Here we're gonna use OnClick because this isn't a form. There's nothing else to add to the cart form.
[00:08:36]
So an OnClick here is the correct thing to do. And we're gonna call this checkout. We need to go provide what checkout is gonna do. But you'll see this kind of passing down of functions from parent to child fairly frequently. You're basically saying like, hey component, whenever something happens, just call me back, right?
[00:08:54]
Just call this function and I'll take care of it. So this cart component is kind of a dumb component, right? It doesn't really have much logic unto itself, it just display stuff. Some people will take this to the extreme and say like there's display components and functional components and they separate everything into display and functional.
[00:09:12]
I think that is silly and I don't do it. But someone does and they are silly. Yeah, so we didn't export default. So that is our cart that seems like it's working okay. The checkout doesn't do anything yet so this will be an undefined function. So if you click it, you're gonna cause an error or we have to add a cart here or checkout.
[00:09:34]
Okay, and looks like I put the cart in the wrong place. Let's go figure out why that's in the wrong place.
>> Brian Holt: But this looks right, I think, so far. So let's go back to order and figure out where this was supposed to be. CSS is easy. I don't know if anyone told you that.
[00:09:56]
This is why this CSS is actually terrible, because it's so reliant on structure to get the correct selectors. Yeah, I just wrapped everything here in an order page, right there and then I put my cart just inside that last div. And that should get everything all laid out nicely.
[00:10:16]
We're getting close here. We should be able to add to cart, okay, no? So if I click add to cart,
>> Brian Holt: Notice that we get stuff that starts getting added, the total is being generated correctly, there's no spaces here, which probably seems like I might have messed up some class name somewhere.
[00:10:44]
Okay, so we're gonna, the cart page, and just after each one of these, we're just gonna add a dash, and that's how I made these like separate a little bit better.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops