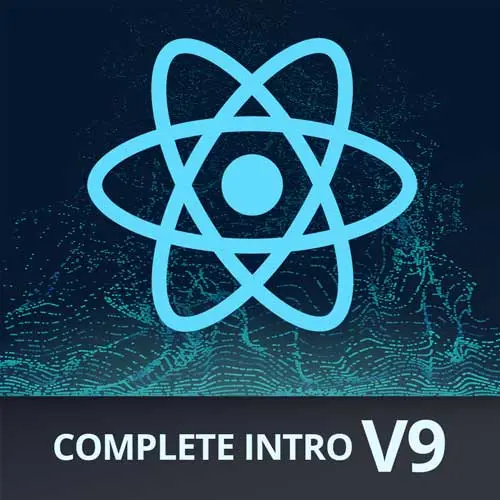
Lesson Description
The "Form Actions" Lesson is part of the full, Complete Intro to React, v9 course featured in this preview video. Here's what you'd learn in this lesson:
Brian installs the release-candidate version of React 19 and refactors the Order and Contact forms to use the action API rather than the onSubmit event. The new useFormStatus hook is also added, allowing children components to see if they're inside a submitted form.
Transcript from the "Form Actions" Lesson
[00:00:00]
>> Brian Holt: Let's do form actions.
>> Brian Holt: So we're actually going to go implement this. So, this is a future disclaimer to people watching this course. If you're watching today with me, just follow along with me. But if you're watching the course, I need you to do something to make sure that you get the right version of React.
[00:00:24]
So go to wherever your server is running or your testing. I'm gonna cancel my testing here. And I need you to do npm info react, and you'll see here what the tag is for, what the current is. You can see the current as of today is 18.13.1. If this says 19, then just do npm install react@19 and you are good to go.
[00:00:51]
So npm install react@19, and react-dom@19 and you should be good to go here, right? If you're following and it does not say that, it says 18 still, just take a look down here at rc. And so mine is here, you can see mine's 19.0. So that's what I'm gonna do today.
[00:01:13]
So I'm gonna do npm install react@rc and react-dom@rc.
>> Brian Holt: And you might even have to force it or did mine go okay? It gives you a ton of warnings because react query a bunch of these things are like, I only support react 18. They take a lot of care to not break stuff, so our stuff should work, but just be aware that you're into kind of dangerous waters now by being on a release candidate instead of something stable.
[00:01:52]
>> Brian Holt: Okay, so just be extra cautious here, or if you don't wanna mess with it, just feel free to follow along with me. So most of web development, in my opinion, is a form, or revolves around a form. It's mostly just taking user data and doing something within kind of being reactive to it.
[00:02:10]
Or, in other words, you really can't get away from doing a lot of forms as a web developer. So the React team kinda took that to heart, and they're like, okay, let's make forms extra easy with react-dom. So, let's just make our mutation function inside of contact.lazy.jsx a little bit simpler.
[00:02:36]
>> Brian Holt: So right now, we have to do this stuff with prevent default and create the form data, and then we can do all this kinda stuff. But let's make this a little bit simpler. Go to your form down here, where I have this onSubmit function and you wanna change this to action.
[00:03:02]
>> Brian Holt: Okay?
>> Brian Holt: And then this is gonna do all of this funny stuff here for you. So you can just delete these top two lines and turn this e into form data.
>> Brian Holt: No, I'm just kidding. Obviously, it's a pretty simple thing. But it's just a nice little nicety.
[00:03:25]
However, we're doing this inside of React query, so we wouldn't do this. But then you get into a function body and you can start doing things like use server in here. And then you can just kind of implicitly handle all the data on the server, and that ends up being a really nice interface to accept data, send to the server, the server then saves to the database, right?
[00:03:50]
>> Brian Holt: That's really quite it.
>> Brian Holt: So, that's it for contact. Let's go do this for order as well. So this is a form as well. So we're gonna go down to our form and have all this stuff here. I think we really only need to grab the set cart out of here.
[00:04:19]
So I'm just gonna grab that and I'm gonna make another function here called function addToCart().
>> Brian Holt: Put that set cart in here, and then this is just gonna be action=(addToCart()).
>> Brian Holt: Again, it just got a little simpler, right? A little bit easier to read, reads a little bit better, so yeah, I'm a big fan of this.
[00:04:50]
Yeah, and to give you a better example of this, imagine I came in here and I had done use server. And now I can do something like sql INSERT INTO cart, blah, blah, blah, and do all of my stuff on the server that inserts into the database. I remember when next, kind of first started talking about this, and people started raging, is like, why are you doing SQL in the browser?
[00:05:18]
I was like, we're not, right? It's okay, this is all happening on the server. But seeing React components together with SQL really just set some people off. It's not just dislike making this a little bit simpler to read, they also do some other stuff that make it a little bit easier to work with as well.
[00:05:35]
So I'm gonna show you one of them. So I want you to head over to contact and we're gonna import the top here.
>> Brian Holt: {UseFormStatus} from 'react-dom', not react, make sure that you're putting the dash dom there because this is gonna forms particular to the DOM, right? They're not necessarily gonna be in React Native, or React-three, or something like that.
[00:06:02]
So that's why this comes from react-dom. And what's cool about this is we have some sort of child component here like this function ContactInput. And we're gonna say return just input. This is kind of a contrite example, but you could see. Imagine you have a input that's a part of your design system and has a bunch of CSS around it and has complex errors and complex validation, you would have some sort of component that does this, right?
[00:06:38]
Using form status, we can actually check to see if the form submitting is pending, which is useful, or submitted. So we're gonna say disabled={pending}, and we'll get that from our form status. We hit name={props.name}. Let's get props coming in here as well. Type={props.type} and placeholder={props.placeholder}.
>> Brian Holt: Okay, and now we wouldn't need this pending thing, and it's really cool all we have to do is say const {pending} = useFormStatus().
[00:07:30]
It doesn't have to have any really concept of where the form is coming from. It can be super buried component, component, component way down in there because React knows where it is. It's gonna say, you're in a form and this form is pending, so you are disabled, right?
[00:07:46]
And now we just have to put this up here. I think you can literally just change this to be ContactInput and type="text". And this one I think I can just literally just say ContactInput, and it's good to go. This will work, right? Cuz now whenever I submit this form, imagine this takes a long time, we don't want people to be typing in there while it's pending.
[00:08:16]
Anyway, suffice to say, this still makes sense and it's still kinda cool that use form status. You can pull that in, despite the fact that this has no concept of where it's being rendered in. That's kinda the point of views, form status.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops