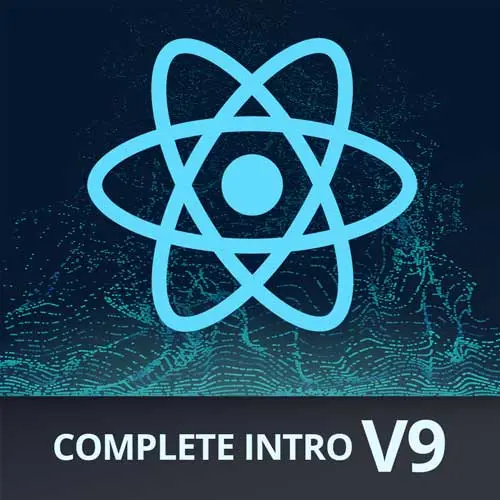
Lesson Description
The "Custom Hooks" Lesson is part of the full, Complete Intro to React, v9 course featured in this preview video. Here's what you'd learn in this lesson:
Brian creates a custom hook to call the `/api/pizza-of-the-day` endpoint from the API server. A custom hook is a function that calls other React hooks. In this case, the pizzaOfTheDay hook calls useState to store the data and useEffect to call the API. The results are returned and displayed in a custom component.
Transcript from the "Custom Hooks" Lesson
[00:00:00]
>> Brian Holt: All right, we're gonna talk about custom hooks, cuz you just hadn't had enough hooks yet. You got to make your own now. One thing that's kinda cool about hooks, is they're composable. So let's say you had, well, let's just talk about the exact example that we wanna.
[00:00:20]
Build here at the bottom of my Padre Genos page, I wanna have a pizza of the day, right? It's gonna be the same pizza every single day, or, sorry, the same pizza every day. It's gonna be the same pizza throughout a day, and then it's gonna change the next day, right?
[00:00:37]
I could write a component that goes in requests the pizza of the day. Day, renders that correctly, blah, blah, blah. But let's say I use the pizza of the day in that particular piece of information seven places in my app. We really want people to buy this pizza of the day.
[00:00:52]
We can write a custom hook that goes and just encapsulates all that logic into one thing. So rather than saying, use state, use effect and all these different things kind of intertwined, we create this one hook that says use pizza of the day, and then it encapsulates all of the logic for you, right?
[00:01:10]
So it becomes this like reusable, composable hook. That's what we're gonna try and build now. So, let's first make our pizza of the day component that's going to display the information from pizza of the day. So create a new component here we're gonna call PizzaOfTheDay.jsx, import, use pizza of, I guess we'll write that here in just a second.
[00:01:42]
But go ahead and write it if you want to, usePizzaOfTheDay
>> Brian Holt: From ./usePizzaOfTheDay.
>> Brian Holt: We're gonna do that same internationalization thing that we did earlier. New.NumberFormat [en-US] because we'll be formatting another price here, style "currency". It's so nice that we don't have to get a library for this that it's just built into the browser.
[00:02:26]
>> Brian Holt: Yeah, okay, cool, and then we're just gonna write kind of a dumb component here. Const, PizzaOfTheDay = an arrow function. And then down here, just make sure you export default PizzaOfTheDay.
>> Brian Holt: Okay. Const PizzaOfTheDay. How many times can I say that in one course? The answer is probably at least a couple times more, = usePizzaOfTheDay, okay?
[00:03:14]
And again, the nice thing is, like, I haven't written this yet, but now that I've done this, I kind of get to approach the rest of the app as just as if it was written, right? Which is pretty cool.
>> Brian Holt: If there's no PizzaOfTheDay, then return some very fancy loading state, which I'm just gonna put in a div.
[00:03:40]
>> Brian Holt: Loading.
>> Brian Holt: And then we're just gonna write this component here. Pizza-of-the-day.
>> Brian Holt: We're gonna put pizza of the Day.
>> Brian Holt: Another div. Pizza-of-the-day-info.
>> Brian Holt: So we're gonna make that an h3.
>> Brian Holt: That's gonna have pizzaOfTheDay.name. P, we're gonna have pizzaOfTheDay.description.
>> Brian Holt: Okay, and we're gonna have pizza-of-the-day-price.
[00:04:57]
>> Brian Holt: And here we're just gonna use, we're gonna say from, right, meaning that it starts at this price, and we're gonna say international.format is gonna be the pizzaOfTheDay.sizes.s cuz the smallest size is always gonna be the least expensive, right?
>> Brian Holt: Div, okay, and then we just want an image of that pizza of the day.
[00:05:34]
>> Brian Holt: Okay, className = pizza-of-the-day-image.
>> Brian Holt: Source equals PizzaOfTheDay.image, and alt equals PizzaOfTheDay.name,
>> Brian Holt: And that should be, good. Now we just got to go write this, but this will display whatever piece of the day that we get back from the API. Beyond that, nothing too interesting here in terms of, components.
[00:06:14]
We've written a bunch of this stuff before, but let's go write our custom hook now. So inside of your source directory, we're gonna create a file called usePizzaOfTheDay.js, you can call .jsx. I don't really care. There's gonna be no direct HTML or JSX rendered. So in theory, you could call it .js I'm lazy and I just call everything .jsx cuz later it might have it?
[00:06:43]
I don't know.
>> Student: It's one of those things, again, like you can make a point for it either way.
>> Brian Holt: Either way, I'm just gonna roll my eyes and say, I don't care and do whatever you want.
>> Brian Holt: Export const usePizzaOfTheDay. Okay, so the nice thing is, like, to make a custom hook, it's just a function that calls other hooks.
[00:07:07]
There's no wrapper for it. There's no additional function that you need for it. Really is just a function that calls other functions, and that's it. That's the entire concept behind a custom hook.
>> Brian Holt: Okay, and we're just gonna encapsulate use state and use effect, like it's just gonna be a combination of those two things, but it's gonna be encapsulated in just like one other hook.
[00:07:36]
PizzaOfTheDay and set PizzaOfTheDay.
>> Brian Holt: We're gonna have this start out as null or undefined or something like that. Again, I would be fine with you putting undefined here, void zero, whatever you wanna put there. My notes have null so I'm gonna put that in there. Use effect and then here we're just gonna write our AJAX request and I'm just gonna put my async function in here.
[00:08:18]
It doesn't really matter where you put it as long as it's in the function body, fetchPizzaOfTheDay. Okay, const response = await fetch/api/pizza-of-the-day.
>> Brian Holt: Man, I would have called this like POTD if I realized how many times I'm gonna get sick of writing pizza the day. Holy hell, await response.json.
[00:08:58]
>> Brian Holt: Okay, and then setPizzaOfTheDay data and then fetch that. How many times do we want this to run? Just once. Just once? What do we put there? MTE, right? You got it.
>> Brian Holt: In theory, if you're doing like pizza drops and people were really pumped about it, you wanted to like change at midnight or something like that, but I imagine people are not that hyped about your pizzas.
[00:09:31]
I don't know, I've waited in long lines for pizza in Seattle, so people do like their pizza.
>> Brian Holt: So again, this is a custom hook. This is a concept that people kind of associate with react, but it's just a function that calls other hooks, right? And this is like my pet peeve when it comes to like libraries and people like to associate these like high mighty concepts and give them like fancy computer science names when in reality it's just a function.
[00:10:07]
Just start calling it a function, right? Because it makes things seem more unapproachable than they actually are, right? Nothing in here is new to you, right? But you probably heard the ideas like, custom hooks, this is gonna be like some amount of formality and I need my monocle and testing harness, right?
[00:10:24]
No, I know it's just a function. That's it, right? And now PizzaOfTheDay works.
>> Brian Holt: Cuz like just mentally model this with me, right? You have this pizza of the day function that you're calling use this, right? Inside of this, this is gonna call two hooks. Now the thing that I told you before is like, don't put them in if statements, don't put them in for loops or while loops or anything like that.
[00:10:50]
But what is this actually doing, right? Inside of it, it's just calling this hook, then it's calling this hook and it's calling them in the same order no matter what, right? It's just in another file, which is okay, and that makes it a custom hook.
>> Brian Holt: Makes it a function, okay, so now we have a piece of the day component, let's go put that in app.jsx cuz we wanna show that to everyone all of the time we are pushing pizza, again, this is intro to capitalism with Brian.
[00:11:27]
By the end of this, you're all gonna be pizza barons. All right, pizza the day there under order, I think is where I put it. Yep, pizzaOfTheDay. There we go.
>> Brian Holt: So let's dev service still running. Make sure your dev server is still running.
>> Brian Holt: Look down here and you have pizza of the Day.
[00:11:58]
>> Brian Holt: Pretty cool, right?
>> Brian Holt: This actually does change, so tomorrow it'll be something different.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops