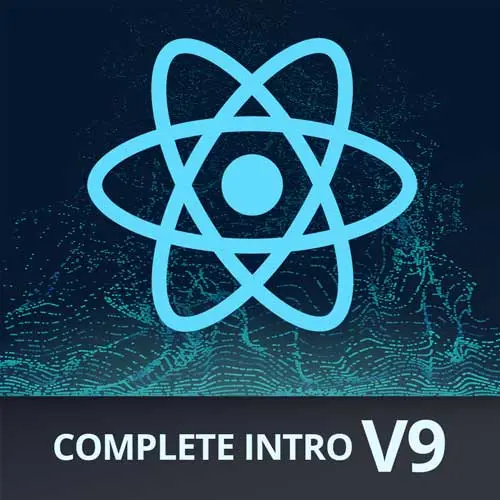
Lesson Description
The "Configuring ESLint for JSX" Lesson is part of the full, Complete Intro to React, v9 course featured in this preview video. Here's what you'd learn in this lesson:
Brian updates the ESLint configuration to work with JSX. The eslint-plugin-react module is installed and a few of the default recommended configuration options are customized. The App.js file is renamed to App.jsx and the App component is refactored to use JSX
Transcript from the "Configuring ESLint for JSX" Lesson
[00:00:00]
>> Brian Holt: So, I'm gonna say npm install-D eslint-plugin-react.
>> Brian Holt: There's just several things that we kinda want eslint to guard against with React, there's just some common patterns. It's funny, I can't even really tell you what they are anymore, because eslint has bloodied me over the years that I've forgotten how to do the bad things, which is probably what you want.
[00:00:26]
Submission by error.
>> Brian Holt: Yeah, that's what we're gonna go fix right now, yeah, Mark.
>> Mark: Apart from class and class name, are there any gotchas when it comes to JSX that you should be aware of?
>> Brian Holt: The other big one that comes to mind is for, right, thinking about labels, right?
[00:00:50]
Label for this input, right? For is also a reserved word in JavaScript for for loops, right? So you have to do, instead of, let's say we had, I don't know, a label here.
>> Brian Holt: You can see it generates it for you, htmlFor, right? And that's just to disambiguate it from for loops.
[00:01:15]
That's the other one that comes to mind that I actually remember off the top of my head. I think there are a couple more, but they're much more rare than those two.
>> Brian Holt: Other than that,
>> Brian Holt: No, there is another one. If you do input, this is valid HTML, it is not valid at JSX.
[00:01:44]
You have to put the self-closing tag on there, it's just how JSX is sure to keep track of things, right? JSX is designed to not be as forgiving as a browser, which I think is generally a benefit, right? So anything that's self-closing just make sure that you put that last slash in there, even though that it's valid JavaScript, it's not technically valid JSX.
[00:02:08]
Okay, so we installed npm eslint-plugin-react@7.37.1 there. Please open your eslint.config. We're going to import a eslint, or sorry, what do we call it here?
>> Mark: reactPlugin from eslint-plugin-react.
>> Brian Holt: Okay, so we're gonna add one more, let's get rid of this, one more config object under this.
>> Brian Holt: And that needs a comma, and we're gonna add our react config into this.
[00:03:04]
So we're gonna say, ...reactPlug in.configs.flat.recommended. Again, spread operator, this is just saying merge these two objects together, give me the union of the two, but we're going to overwrite the settings of it. And we're gonna say react:version,
>> Brian Holt: detect, this one kind of annoys me, it is necessary, I don't know why this isn't the default.
[00:03:44]
The eslint plugin wants to know the version of React that you're working with, that tracks, right? It makes sense that you would want to be linting for version 19 versus version 16, they're quite different, right? There's some difference there, and they have a really cool feature. It's like, hey, look at my package.json and use that, okay?
[00:04:04]
Just always do that, right, that's always what I want you to do, there's never not the time that I don't want you to do that, anyway. Thank you for writing free and open-source software that I get to use, but I'm cranky and I don't wanna do that every single time, but you do.
[00:04:19]
Anyway, this is what you have to do every single time.
>> Brian Holt: So [COUGH]
>> Brian Holt: Yep, and we need one more here underneath that, we need a reactPlugin.configs.flat, one called jsx-runtime.
>> Brian Holt: This one is the one that will fix the error around not importing React, right? Cuz otherwise, it's gonna be like, I expect React and React is not here.
[00:04:57]
Make sure you added jsx here if you did not previously, otherwise it will not lint your jsx files, which is kinda the damn point. And then, after language options here, let's add some rules. Oops, yeah, rules.
>> Brian Holt: The reason we put rules here and not in one of these other two, otherwise, you'll overwrite their rules configs.
[00:05:32]
These new eslint configurations are tricky, right? So, anyway, the order becomes extremely important. So, react has two really annoying default rules, and we're just gonna turn them off cuz I don't wanna deal with it. No unescaped-entities.
>> Brian Holt: Yeah, it forces you to do weird, it wants you to directly escape hyphens and ampersands in your code.
[00:06:10]
I don't wanna do that, I want my tools to do that for me. So I turn that off always and then react/prop types. I don't know why this one still exists, there used to be a thing called prop-types, that as soon as TypeScript became a thing, just instantly went away as being useful at all.
[00:06:27]
But it's still the default that it's on, I don't get that either. So, just to drive home, if you don't have this, you can't write single quotes inside of your strings, you have to write, what is it? And apos, right? You have to write that instead of writing that.
[00:06:46]
And after doing that twice, I was like, yeah, this shit's for the birds, I'm not doing it [LAUGH]. So, that's why we're turning that one off.
>> Brian Holt: Okay, I think we can leave eslint alone for a while now. Yea, if you're wondering about flat, that's the new style of configuration for eslint 9.
[00:07:10]
These are flat configs, which is where that flat word comes from. Okay, let's go convert app.js now. So first thing, rename this file to app.jsx.
>> Brian Holt: Okay, we're gonna delete the react import cuz we talked about that. Import pizza from pizza, we did that and let's make this a bit more nice-looking app.
[00:07:47]
>> Brian Holt: Okay, return,
>> Brian Holt: div,
>> Brian Holt: Each one, we're gonna call that Padre Gino's. Order Now, I could just do that in normal and so it doesn't highlight it.
>> Brian Holt: And then we're gonna do a pizza component,
>> Brian Holt: Like this, name=Pepperoni.
>> Brian Holt: Description=,
>> Brian Holt: Pep, cheese, stuff, again, self-closing.
[00:08:43]
So one thing to call out here, notice that this is h1, lowercase, h1. Notice this is P, pizza. I don't do this just by convention, it's actually required in JSX and React. The lowercase means this is literally a DOM tag that I would like you to render just like this, right?
[00:09:05]
So if I have x, my web component,
>> Brian Holt: This means it's gonna expect to actually render that because this is lowercase.
>> Brian Holt: Whereas the P, let's this knows like this is actually a synthetic one that I've created, right? And so, you have to name these uppercase. So, one is both useful just from like at a glance to be able to tell what's a DOM component versus what's a React component that I've created.
[00:09:39]
And it's required by the tools.
>> Brian Holt: You can see, just like you give this, like a class name or something like that, right? You can pass in properties, just like as if they were like DOM properties. It's meant to mimic kind of the same kind of way of doing it.
[00:10:01]
>> Brian Holt: Okay, let's make a few more of this,
>> Brian Holt: Hawaiian,
>> Brian Holt: ham, pineapple.
>> Brian Holt: And this was,
>> Brian Holt: french fries.
>> Brian Holt: Okay?
>> Brian Holt: Then this one down here, instead of having React.createElement(app), we're just gonna do app, like that, okay? Save that, one more thing to do, go look in your index.html and we renamed the file, so we have to re-put the x there, right?
[00:10:57]
See if you're gonna run our project now, npm run dev, have this open Padre Gino's.
>> Brian Holt: This was wrong, so 5173, and you can see, everything still works.
>> Brian Holt: Pretty cool, right?
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops