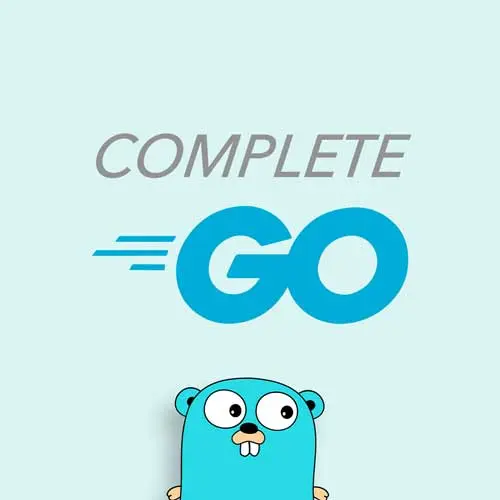
Lesson Description
The "Variables & Basic Types" Lesson is part of the full, Complete Go for Professional Developers course featured in this preview video. Here's what you'd learn in this lesson:
Melkey introduces variables and some primitive data types. A variable can be declared with a type, or the type can be inferred based on the value. Format specifiers are used to print formatted values to the terminal.
Transcript from the "Variables & Basic Types" Lesson
[00:00:00]
>> Melkey: So, we have this Println, we can just remove this, again, the compiler is gonna be yellow, that's okay. We're gonna start off with variables and variable declaration, cuz there's a couple different ways you can do this. First of all, this how you write a comment, I'll just say variables, right?
[00:00:15]
Comments are just like slashes, you can do other variations of comments, but it's in line, does the trick. So the first method of declaring a variable is using the keyword var, the name of the variable, and then the type of the variable. Or it's gonna be a string, and we can say this is gonna be Melkey, okay?
[00:00:35]
The compiler is once again yelling at us, cuz we have unused variable. So if you have any unused variables in your code, you will not be able to compile, you won't be able to run your project. So again, unused imports, unused variables, we don't like that in Go.
[00:00:50]
To print this, instead of using Println, which is the first print statement I used when we started this, I'm gonna use something called Printf. And Printf formats according to a format specifier and writes to standard output, okay? Now, what that means is it's gonna first take what we want.
[00:01:06]
So the template string here, I'm gonna say, this is my name, and then the formatter, right? So different data types have different formatters. A string is modulo s, I might say percent sign s, or modulo, I am referring to this symbol right here. Key distinction between Printf and Println.
[00:01:27]
Println gives you a free line, Printf does not. So you have to specify the line break with /n, like so, okay? And then with Printf, the arguments are going to come in into the order they appear in the template string, from left to right. So here we have one, it's gonna be a string, and pass in our name, like so.
[00:01:47]
We can save this, and we can just quickly run, go run main.go, and we should see this is my name, Melkey, all right? So that's one method of declaring a variable. Another is Type inferencing, so the compiler can actually inference a type for us. The way you can do this, let's say we have an age, let's say the age is 27.
[00:02:10]
You can see here, it looks vastly different than the var name, but this is still a variable, but now the compiler is inferring the type for us. It's gonna infer that 27 is a type int, okay? So we can do the exact same thing, fmt.Printf, okay? We can say this is my age, and then the format specifier for an int is modulo D.
[00:02:31]
Again, do not forget the break line, and we can put age. Okay, now if we save this, you can see this is my name, Melkey, and this is my age. So, Go has the ability to infer, and we can do the same thing with name as well. We can just do name with the shorthand operator, okay?
[00:02:48]
I think this is called the walrus operator in Python, to declare something and have Go infer type for us, okay? Another method is to declare but reassign later. So we can do var, keyword, the name, city, and then the type, string. And we can just leave it like this.
[00:03:08]
This is fine, so, different than the first variation, we didn't explicitly set what city is. Cuz the compiler is only yelling at us, hey, it's declared and not used, what's going on? So we could do something like this, and then we can say city = "Seattle", all right?
[00:03:24]
And again, we can do the exact same thing, fmt.Printf, we can say, this is my city, okay? Format was the module operator, and say, city, like so. You can now see we have Melkey, age, Seattle. If you wanna declare multiple variables at once, you could do something pretty easy.
[00:03:46]
You could just do var country, and then comma, and you can do another thing, like continent. Call these strings, and just equal. And then you can set them in the order they appear, so you can do, USA, oops, and North America. Okay, declared and not used, so this is a different way to just specify the same type.
[00:04:07]
We can do fmt.Printf, this is my city, and then module s, and this is continent, okay? And then in the order they appear, so put country, and then continent, like so. It's going to keep going back and forth, go run main.go. You can now see, I said city, whoops, sweet country, yeah?
[00:04:37]
Can you declare variables of different types? Yeah, [INAUDIBLE] Not exactly like that, but very similar. So if you want to declare different types, you can do var, and then open it with these parentheses. Okay, and this is where you can put a list of different types. So let's say we have one called is employed, I'll make this a Boolean.
[00:04:57]
We can set this to true, okay? And then let's say we also have a salary, all right? We can do salary of type int, we can set this to like 50k. So, 50, 1, 2, 3, we can save this, okay? And then we can have one more, that's position.
[00:05:13]
Position, which would be string and we'll just say, developer, okay? And you should notice, if you save, depending on VS Code or whatever, you should get that auto formatting from Go font, gofmt. So, double check if you have that working. All right, so this is a way to declare different variables, different types, all kind of, in line, quote unquote, cuz it's multiple lines, but you know what I mean.
[00:05:39]
And again, can just do fmt.Printf, you can say, is employed, the formatter is going to be T for a Boolean, this is my salary. Modulo d, and this is my position. Okay, and in the order they come in, so we put isEmployed, salary, and position, okay? And I'm gonna do this one to show you something.
[00:06:15]
So if I run Go, run main.go, you can see we have everything, minus the typos, right, working, but you see this little percent thing at the end, right? That's because I forgot to put the break line, or a new line. So if we were to do another print statement, it would just append directly where this character is.
[00:06:35]
So that's where we have the break line, or you can use Println if you want. Someone may have noticed that we have this interesting one right here, I wanna put a focus on this. So we have var city string, var city string, okay? And we did assign it, so in between this line right here, and this one where we assign it, what is the value of city, what is it, right?
[00:06:58]
Compiler's not yelling at us, it's yelling that, it may not be on use, but at the time of writing it, it's valid, the question is, what is this thing, right? So, there's a concept Go has called zero values, okay? Also known as default values, you can use whichever.
[00:07:18]
And I'm gonna demonstrate these with a few different types here. So let's say we have var default int, I'm gonna set this as an int, var default float. I'm gonna set this as a float, either 64 bit or 32 bit, we'll do 64 bit. We can do var default string.
[00:07:36]
Okay, and then we can do var default bool as a type bool. All right, so we have these four different variables, all with this zero value associated with them right now. So let me ask you guys, if I were to print these, what will be printed out? If you were to guess just randomly, what will be printed out?
[00:07:58]
000, false. 00, okay, so for the first int would be 0. Sorry, yeah, 0, 0.0, empty string, false. That's 100% right. So these zero values is how the compiler basically assigns us that this is going to be an int, or a float 64, or a string, or a bool, right?
[00:08:21]
Now, that's pretty helpful, right, it kind of avoids us having that nil error, potentially. The downfall of something like this is when you wanna pass this as data, and you want this data to have a very specific identity, or specific value associated to it. So like you said, right?
[00:08:42]
The default bool is gonna be false. Well, booleans can only be false or true, right? So it has to be one of the other, cuz it has to be one of those default values. But let's say you're passing data and it's neither false or true. Let's say it just doesn't exist.
[00:08:57]
Let's say there's a form, right, that we are accepting, that's JSON. And someone omitted a check that says that they are either this or this, or whatever condition you can put for a boolean decision. And it did not set it, so what is it, are we gonna use the false value by default?
[00:09:14]
Or do we put it as nil, right? As if we don't know, it's undefined. So that's one of one of the drawbacks of having these zero values. They're very useful, and they avoid potentially bigger issue. But there is a drawback where sometimes it's not an empty string, sometimes it's not there, right?
[00:09:32]
An empty string and just not having a value are two different things, right? So there is a bit of a difference there. I'm gonna quickly just print these, oops, fmt.Printf. I'm gonna do just int: float module d, float module f, and then it's string. In the order they come in, so default and float.
[00:10:11]
Cool, oops. All right, let me make sure that works, cool.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops