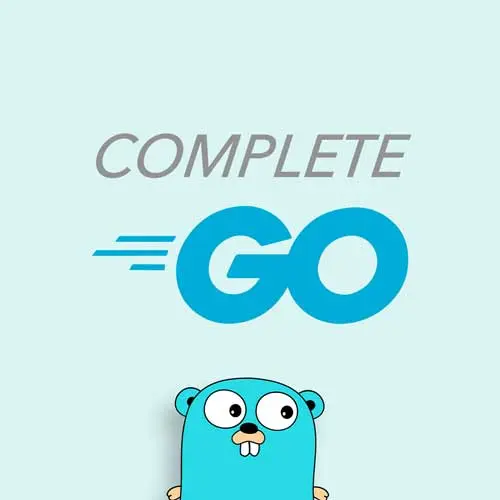
Lesson Description
The "Validating User Data" Lesson is part of the full, Complete Go for Professional Developers course featured in this preview video. Here's what you'd learn in this lesson:
Melkey adds a function to the user store to validate the incoming request. Conditions check each property to ensure it exists and is formatted correctly. A RegEx example validating the email address is also included.
Transcript from the "Validating User Data" Lesson
[00:00:00]
>> Melkey: With the database layer done, let's go back into the API. Let's go user_handler.go. And we're gonna mimic the same behavior we had with the workout handler, but now we're gonna have it with the user handler, okay? So first thing, let's go ahead and create the package API.
[00:00:17]
Next, let's do a UserHandler. It's gonna be our struct, this UserHandler needs to have two fields in it. One is going to be the UserStore, so store.UserStore, which are interface and the second thing is the logger, which is going to be point to log.logger. Right, underneath, let's go ahead and create our constructor, so func NewUserHandler.
[00:00:42]
This is gonna take the UserStore of type store.UserStore, and it's also gonna take a logger, pointer to log.logger. It's gonna return a pointer to UserHandler like so. In the function, we can just return the address of UserHandler, like so, user store is gonna be UserStore and logger is gonna be logger, all right?
[00:01:06]
And something different I'm going to introduce into this section is, we're gonna have, again, remember we had that validation request from updating a workout. We're gonna extract that over here, so we can do something like type registeruserrequest, this is gonna be its own struck, like so. It's gonna have its own field, so we're have username, we can do some like string.
[00:01:32]
You can have them as pointers, I'm not gonna use points for here because this is for specifically registering a user, so these are fields we want to assure our mandatory, right? There's a bit of a difference between creating something and updating something. For the update, that's what we had those pointer types to check if they are nil or not.
[00:01:52]
Just again, because it's go the zero values could be a bit of a gotcha, but because this particular struck is going to be used for creating a user, we can kind of take them more at face value and not have that validation. Again, you don't it's just added protection, if you want, you can definitely add a pointer and do those same checks here, but since it's already implemented in a previous file, I'll leave that up to you to use if you like it or not.
[00:02:18]
So here we have username string, we can just continue on with the JSON. So we can put username, like so, we can have our email string can be, oops, JSON email. Okay, we're gonna have our password. It's gonna be a string. Again, this is the password that we get from the caller, okay, we do password, and then we can have bio, which is string.
[00:02:45]
Let's read bio, like so, okay, now for server side validation, like I just finished talking, we don't have those pointers, and we don't really need the pointers. However, we can still add some sort of function to validate, is the email properly formatted? Is the password, less you can't put a password one character, right?
[00:03:10]
So we can have those validations on our file here, again, you can have them in the front end for client side validation. I don't see a problem having them on the back end for backside validation as well. And I think it's just useful to demonstrate how this could be done.
[00:03:27]
So we'll just do a method on our UserHandler. We can call this validateRegisterRequest, okay? And this is going to take a pointer to that new register request. Just struck that we've created and it's going to return an error or no errors, okay? And here, it's pretty simple, we can do ifrequest.username is like, empty, for example, right?
[00:03:56]
We can say return errors dot, oops. So we've seen this when we looked at the error SQL, new errors, we can say username is required.
>> Melkey: Okay, and we can create this new errors using the built in errors package and go, so errors.new, and here you can go nuts with all of the constraints that you want.
[00:04:17]
The username length, the username, if it's too short, too long, you can add them here, I'll show one example, right? If length, sorry, if lengthrequest.username, let's say this is greater than 50. Well, this can be an error. First, we return errors.new, we can say, username cannot be greater than 50 characters, something like that, right?
[00:04:48]
So however you wanna handle usernames is totally up to you. We can do the same thing with email. So ifrequest.email is empty, since it's a mandatory field in our database, we can return errors.New. I'm going to say email is required, okay? And I think a more interesting one, which may require me to push and you guys to pull down in just a second, is the regex component of an email.
[00:05:17]
So go has a standard library a Standard Regex library, and it's pretty good. It's a pretty good Regex library, I'm not gonna try and claim, I'm like a Regex master, I can't read Regex, I don't believe people that can read Regex, I don't believe them, they're wizards. But, essentially, it doesn't hurt us to also have a validation for Regex, okay?
[00:05:39]
So here, we can do something like email regex. We can bring in the regex standard library, so regexp. I can use the must compile function here, and this accepts a string. I'm gonna particularly use backticks. Now, I'm gonna copy and paste what this is, I'm gonna push the code up.
[00:05:59]
So here, I'm gonna grab this.
>> Melkey: And this is the formatted for an email in regex, according to Google. So continuing on, we can say if emailRegex, right, if it's not matching these strings, so this method, right here, match string into the request.email. Okay, so if this is false, meaning the email does not match the regex email formula we have, we can return errors.new, and we can say something like invalid email format, okay?
[00:06:42]
And so, again, we can imagine this like, we can have password validation as well.
>> Melkey: I can quickly just show you one, but I think it's fairly obvious what what the thing is you can do ifrequest.password is empty. You can see return error.New password is okay and yes, I agree that this would probably and should be done with the front end.
[00:07:15]
But what if your front end is written by someone who doesn't know what they're doing? Or what if you wrote the front end like a 3 AM when you're buzzing on Red Bull, jewels, Zins, everything the devil's on your shoulder. Like, it's easy for things to slip right up because it's said to be done on one side of your application, doesn't necessarily mean it's, a, done correctly, or b, can have negative consequences on your back end.
[00:07:38]
And further strengthening my point, stuff slips on the front, big whoop. Your form is gonna miss the value, okay? Here, if we accept values and persist it into a database, that's big trouble, that could actually have some some consequences, right? Now, our database is, I won't say the word infected, but it has improper data.
[00:07:59]
Yeah, so that's why I always, personally like to add the extra stuff of my own back end validation. This is not gonna cover all the cases, but what it does try to do is validation that you see in on the front end. You can easily copy it on the back end as well.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops