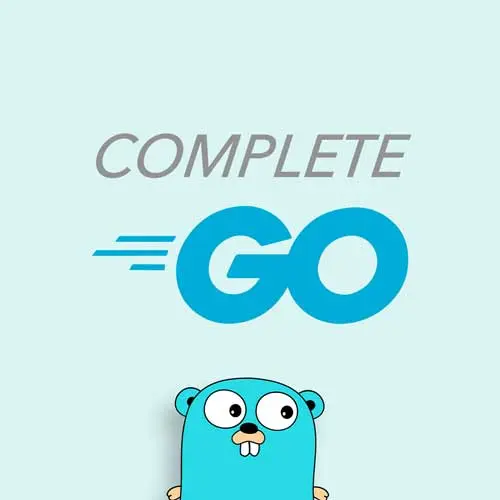
Lesson Description
The "Slices" Lesson is part of the full, Complete Go for Professional Developers course featured in this preview video. Here's what you'd learn in this lesson:
Melkey explains that Slices behave like dynamic Arrays. They are not bound by a predefined size and can grow as more items are inserted. The append method adds values to a slice. This lesson also demonstrates using a for loop and the range identifier to loop through a slice.
Transcript from the "Slices" Lesson
[00:00:00]
>> Melkey: With arrays, they're fairly defined. Then there's slices, okay? And so the kind of two hand definition of a slice is, slices are dynamic arrays, all right? So when you think of an array in Python, that's kind of what a slice could be, right? And what's happening under the hood is a slice is defined.
[00:00:19]
With a certain set size right, that we don't define it. The com, the memory compiler understands it. And then when we wanna add more valleys to our slice, what's happening is the Go compiler, Go code is actually just doubling it every single time, okay? So that's how it copies that dynamic nature.
[00:00:37]
An array, it's very rigid, it's defined. It can only hold five ints, and that's all it could do. And it's your choice to use them or not. Whereas a slice, you can define it as anything like it could be five slice, it could be five values, and you can append more to it.
[00:00:51]
You can continue to append to continuously grow pros and cons arrays more memory efficient, more memory, you know, good for better for the memory cuz the compound knows right away how much allocate a slice changes on the fly. So some key distinctions there. And another definition of a slice is a slice could be a slice of an array, so a portion of the array, right?
[00:01:17]
If we defined our array as the full five slot, a slice could be a portion there, or in some cases, could be the entire thing that you wanna make more dynamic. Let me show you an example. So above, we have our numbers array, right? We can have something like all numbers.
[00:01:34]
We have a short form notation. We can say numbers with this syntax here. This is basically saying we are making a slice copy of our numbers array, and now we have all numbers, okay? So at this point in time, all numbers and numbers are both data structures that are hold five integers, 10, 20, 30, 40, and 50, okay?
[00:01:58]
The key difference, however, with all numbers slice is now we can append more values to it, and we do not adhere to the constraint of the array. You can also do this a little bit more explicitly, with first three as numbers, and we can just bring in the first three values, so we can index the zeroth to the third value here.
[00:02:22]
And this is how it's like a slice of the array. It's like a portion of the array that we're going to use, okay. And so we can have different methods on it. We can do all numbers. And you can even see here we're getting this nice little hint for all numbers right with this append key function here which if you notice if I type in numbers does not exist at all, okay?
[00:02:43]
Arrays do not have the ability to have values appended to them. Slices can, and what's happening under the hood? Don't fully call me on this, but depending on how much size being allocated to the slice, Go is going to double it in size every single time, right? So if you want a slice of three and it starts off as a slice of two, goes gonna double it.
[00:03:05]
So your slice is gonna have a capacity of four. And next we add two more values, gonna double it and continue so forth and so forth, yeah?
>> Student: Is it like a linked list or something, or is it actually just creating a new array with twice the capacity?
[00:03:19]
>> Melkey: It's creating a new array of voice capacity. Check these things out. This is a really good case of a slice. However, I think a more practical, less like, I'm gonna teach you something course feature of slices is using them as dynamic arrays. Okay, so for example, let's say we have this fruit slice.
[00:03:41]
The syntax to declare your own slice is very similar to the syntax to declare your own array with the square brackets, but this time, that's it string like so. So the key difference is we do not have the capacity in the notation here, it is just indicating to go.
[00:04:01]
Then now this is going to be a slice instead of a rigid array. This, again, I was saying this pros and cons to this right memory allocation speed but see how it is with more of the use case that those data structures offer. If you know the size of the data be handling is going to be five and is no need to use a slice, use an array.
[00:04:24]
Makes more sense you don't lose anything. If you know your data could be growing, shrinking, going diagonal, I don't know, use a slice or yeah, use a slice. The other one use an array. For fruits, we can have apple, banana, and I don't know, strawberry, right? So now we have our defined slice of fruits.
[00:04:46]
We can do format, print F, these are my fruits. Modulo V, break the line and type in fruits, right? You can see now we have apple, banana, strawberry, which is great for a smoothie. But now we can explore some built in methods that really give a good appeal to two slices, right?
[00:05:08]
So here we can do something like fruits, and we can use the built in keyword append. So if you wanna append something to a slice, you have to do append, then the existing slice as it is, as the first argument to the append function, and then the value you want to append to it.
[00:05:25]
So let's say I want to append a kiwi. This is great, this works if I now print that same these are my fruits with kiwi. You can see now we have apple, banana, strawberry kiwi because we've appended to it. We can look at more things. You can actually go a little bit more intricate with the append method.
[00:05:46]
So we have like append fruits and you can append like a bunch of different things too. There's not gonna be just one. You can put like mango, pineapple, right? So from like a use case perspective, it's very convenient with more fruits, right? But under the hood, like what's happening with the append function is like, we take the size of the fruits array as is, we look at how many things we're appending into this append function, and then we either create the new slice, or we use the existing one with the existing capacity, right?
[00:06:16]
So now, if we just run this, we can see now we have apple, banana, strawberry, kiwi, mango, pineapple, we got all of them. And you can even append two slice together. You can have, like, more fruits, do the same syntax right here, string, I actually don't even know blueberries.
[00:06:36]
What's another fruit, tomato. And then you can do fruits, append fruits and then more fruits. And you can also append it with this syntax here for using all of the fruits in the more fruit slice. And if you run this, yeah.
>> Student: Does each append create a new slice?
[00:06:57]
>> Melkey: No, not necessarily, like I said earlier, so we're, what happens under the hood with slices in particular, Go will double the size of the slice. So if you go from a size of two, you double it, it becomes four. If you wanna append one method to it, you will append it to the newest one, right?
[00:07:18]
So now you have three values and you have this open size under the hood open size of your slice. So if you add one more value, it becomes fully four. But if you add more, that slice then gets doubled to eight, and you can add up to four new values before it gets doubled again.
[00:07:32]
One thing I want to show also, before we move on to maps here is, we have arrays, right, or a slice. Let's just go with the arrays. Our numbers are if you go back, it's 10, 20, 30, 40, 50. When I showed you the first for loop with for i equals zero and the condition of increment it, that works great.
[00:07:49]
But if you wanna iterate through like a range or iterate through us a for loop, a slice an array, you can do something using the for loop you can do for index and then value. In range, so the range keyword numbers, okay? And this is just a short form notation to iterate through all the values in an array or a slice or any kind of object, right?
[00:08:13]
And you can also do format print F. This is, or if you just say index, module D, and value, the modulo D.
>> Melkey: That's right, so we have index 0, 1, 2, 3, 4, 5 with the value associated to it.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops