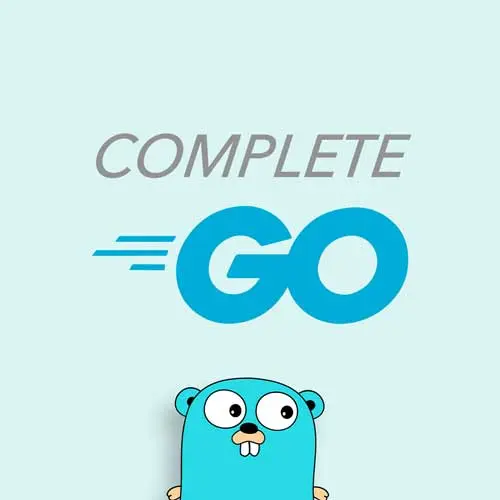
Lesson Description
The "Functions" Lesson is part of the full, Complete Go for Professional Developers course featured in this preview video. Here's what you'd learn in this lesson:
Melkey creates a few functions to highlight some unique language features in Go. Functions can provide multiple return values, and parameter types can use a shorthand syntax with the last parameter's type being applied to all the preceding parameters.
Transcript from the "Functions" Lesson
[00:00:00]
>> Melkey: I wanna quickly jump into functions cuz I know we're writing into a function, but I kind of cheated the explanation of it because it's the func main, so I kinda just wrote it. I was like, yeah, and I kinda just skidded my words. Let's kinda take some time to look at the function signature in Go.
[00:00:14]
So I mentioned earlier, it's the func keyword. Every function is defined with the func keyword. There is no pub or public predecessor in Go. If you wanna make a function public, you'd capitalize it. I'm gonna express and go into some of those gotchas. But for instance, if I call this function, add, and I do A-D-D like this, this is not exported.
[00:00:40]
Even if it was in an exportable package, it cannot be exported. If I only change this, it is now exportable. Well, I'll show you in real applications of some gosh that this leads to. But for our case, we can just go ahead and make a quick function called add, then it's gonna be the parentheses, and within the parentheses we're gonna take the arguments for our function.
[00:01:04]
These are separate with comma so we can have a, b, okay? And then here it's the return type of our function. So is our function return int type, string type? Ours is going return an int and then the curly braces define the function. Now, there's a few compiler errors right away.
[00:01:21]
One up here says a and b are undefined, and that is because in every argument declaration in this parentheses, you have to specify the type as well. So in this case, a is gonna be an int, and b is also going to be an int. And then we have the missing return, so the compiler, if it knows that it's gonna be a return type from a function, it's gonna expect it.
[00:01:44]
If I simply just remove this, that error goes away, okay? So we can go like this and we can simply return a + b, okay? So that's the very simple definition of functions in Go, name, arguments with the type specification, the return type, if it does exist, and then the actual logic of whatever goes down in the function itself.
[00:02:07]
If we wanna use this function, we can simply just do add and then put 3, 4, right? Now, if you see this, is there anything that looks a little off about this?
>> Speaker 2: The return's not being caught anywhere.
>> Melkey: Yeah, exactly. So we could use add(3, 4) like this as the actual return and just send it out.
[00:02:29]
I mean, you could definitely do that. But in Go, you can actually omit the returns entirely, point blank. If this function had five different return types, we could ignore four of them and be fine with it, right? There's a way you can ignore them. And this falls into conversation of error handling because in Go, errors are values.
[00:02:52]
I'm not gonna demonstrate it right here. You'll see the example of errors when we create a function that actually handles an error. But you can see how that could be a potential foot gun. So here in our case, we just do result. We can do the shorthand notation to declare that add is gonna give us the result, all right?
[00:03:08]
And we can print this, the format, we'll use just print line. We can say this is the result. We can just paste result like this. If we save it and we flip, you can now see this is the result, 7, all right? Nothing too crazy, but let's say we wanna make another function that returns two return types.
[00:03:31]
We could do calculate sum and product. We can go ahead and take a and b in a nice little syntax sugar. It's starting a and b int. If you know they're going to be the same type, you can just do int at the end to cover the grounds of all the arguments before that int declaration.
[00:03:48]
And if you want your function to return more than one type, you have to specify it with a parentheses with these brackets here, and you can do int, int, right? And then we can specify the logic, this can be return oops, a + b, a * b, like so.
[00:04:09]
So one return statement separated by comma to distinguish between the two different types that we expect this function to return. Then here, we can just do something like sum, yeah?
>> Speaker 3: Do you have multiples? If you had a, b, those are both ints.
>> Melkey: Yep.
>> Speaker 3: Is it just like everything to the left if you did another type?
[00:04:28]
>> Melkey: Yeah, so let's say a, b, and then let's say the next one's gonna be a float, f would just be float64 bit, and that's fine.
>> Speaker 3: And you can put as many before that?
>> Melkey: Yeah, exactly, we could say name, string, continue this. If you want name and let's say, I don't know what else could be a string?
[00:04:48]
Tree, I don't know, this is first thing came to my mind, this is all valid. The only thing that the compiler's gonna yell at us is it's just unused parameter, but this, it will still compile, it's just gonna tell you that you have unused parameters in your function.
[00:05:04]
>> Melkey: Okay, so if you wanna get multiple returns from that function, we can do something like sum separate by product or comma separating product. Same kind of shorthand notation to calculate sum and product, let's do 10, 10, fmt.printf("this is sum %s and this is product %s\n", sum, product").
[00:05:35]
Save this and, sorry these are not strings. That's an example of the specifier. These are supposed to be integers, like so. So this is the error you get. This looks really bizarro. If you run this, that works.
>> Speaker 4: When you're returning multiple values like that, is that automatically de-structuring a two-pool or something, or something or?
[00:06:01]
If you just stored the result of that, calculate sum of products into one variable, is that a different data type you can work with or is that not even valid?
>> Melkey: Sorry, what do you mean?
>> Speaker 4: In Python, if you were to return two values like that, it'll be returned as tuple.
[00:06:16]
>> Melkey: Yeah, these are not tuples. So what you can do, so this is not gonna be considered tuple because like I said earlier, you can just do something like this, and now we can completely omit the return type from our calculateSumAndProduct. Now we just have sum that we deal with.
[00:06:30]
The compiler's mad at me cuz we don't know what product is, cuz I removed it, but this is completely valid.
>> Speaker 4: So multiple returns are like a kind of first class idea?
>> Melkey: Exactly, yeah, so every variable every return type is handled as a first class citizen that you can do whatever you want with.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops