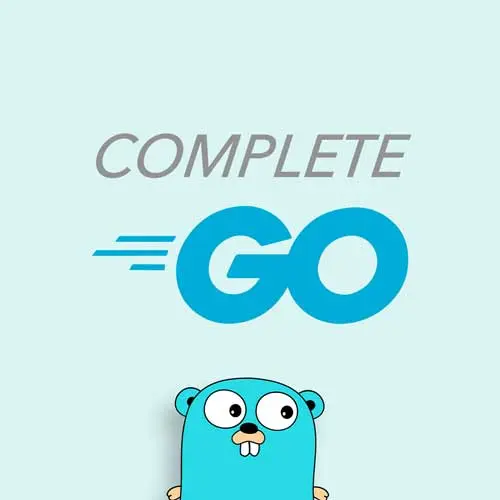
Lesson Description
The "Course Overview" Lesson is part of the full, Complete Go for Professional Developers course featured in this preview video. Here's what you'd learn in this lesson:
Melkey walks through the course outline and shares details about the application that will be built. This course will create an HTTP server and a complete CRUD application for creating and managing workouts. It will include an authentication layer and robust unit tests. The tech stack includes Go for the backend language with Postgres running in a Docker container.
Transcript from the "Course Overview" Lesson
[00:00:00]
>> Melkey: I wanted to provide everyone with all the tools that they would need if they were to go back and write go on their own. We'll write HTTP Servers using Go, we'll write Unit tests in Go. So we'll write pretty intense tests as a whole section of it.
[00:00:17]
We'll obviously learn how to connect and write to our database. We'll learn to connect and write to our API, Stella Write and Go. We'll also have handlers for user interaction, so imagine having users, right? Imagine building an app and actually having users, we wanna give them a proper experience.
[00:00:35]
We're gonna show them we can handle their information, that their information safe, that it's able to communicate through everything else in the app. So we're definitely gonna have a core part of how users will work in a back-end setting. And then for that, we'll have a middleware, we'll create our routing.
[00:00:53]
I think middleware is actually a really cool topic that shows how we can inject something into the request or the response, and how we can really use it to our advantage. Who does this course benefit the most? I think that's probably an important question to ask, and it's really simple, kind of going back to the objective function of this course.
[00:01:14]
And it's anyone who's interested in learning the Go program language. And again, that's kind of it, I can move on to the next slide, but I'm not going to. It's a very simple kind of mission statement, if you think about it, because it covers a lot of ground, right?
[00:01:28]
If you someone who's a junior engineer, right? Let's say you're very green, out of school, you're just learning it, and you maybe tinker around with JavaScript, or TypeScript, or Python, one of those first languages that introduces people to programming. I think Go is a great first language to learn, I truly think when we think about first programming languages, Python, JavaScript, are one, two, I don't see any reason why Go is not in that conversation.
[00:01:54]
I actually would make the argument that Go is probably better than the other two. Because if you wanna make a solid career as an engineer, as a programmer, as a developer, Go will expose more elements of computer science than JavaScript and Python, right? It's just more intricacies about Go, I'm not saying it's the lowest level implementation because when you go lower the barrier to entry for a new person is kind of tougher, right?
[00:02:22]
You're not gonna really recommend someone brand new, something like C right? Or Rust, or maybe you would, but I think a lot of times you do that, there's a chance some people may get discouraged, right? Because programming is hard, especially if you're new, I don't know if you remember the first time you wrote code, you're probably I don't like, what, I don't get how this works.
[00:02:42]
And so I think Go it's a very subtle way to get introduced to programming, and it introduces quote, unquote advanced intermediate topics that Python and JavaScript kind of abstract. But it's also different people's experience, so let's say you're a mid-level engineer and you've been mastering your tools, which could be web dev, or could be something else.
[00:03:06]
But you wanna make a transition, right? You wanna go from front-end to back-end, you sometimes pique your interest. Go, again, is a great language to seek out in that endeavor because I think other languages may have different criteria for use cases, right? Maybe have different boiler plates attached to them, which makes everything kind of overwhelming.
[00:03:29]
I think if you're first kind of interested in back-end languages, and you see if it's truly something for you, I think Go is a great language that does that. And then even for seniors, even if you're a senior engineer and you've been mastering Go for a long time.
[00:03:42]
I do hope there's going to be things in this course that either remind you about certain topics of Go that maybe you've forgotten. Or maybe you choose something new that you didn't even think of implementing in your own projects. So I truly hope this covers a wide array of people who want to learn Go, or going out of their way to learn Go.
[00:04:02]
Or maybe have heard of it, have this kind of itch, and then hopefully this course, scratches that itch. So finally with all that yapping, we're gonna talk about, what are we going to build? So we are going to be building a workout posting service. In our project, we're going to give users the ability to create accounts where you're gonna have people that can sign up.
[00:04:24]
We'll talk about different authentication methods, cuz I think there's a few more popular ones I think a lot of people may know of already. And I think there's some that maybe some individuals aren't aware of. And I did a bit of a deep dive in authentication methods myself, and this section here where I talk about different methods and which one we're going to use.
[00:04:49]
But yeah, we're gonna allow our users to create workouts, we're gonna give our users the ability to edit their own workout, that's a key, distinguish the quick key part. We don't want someone to create a workout and someone else edit that workout, that's obviously not something we want.
[00:05:04]
Users can delete their workouts. Again, I wanna create a workout, I don't want someone else to delete my workout. We can retrieve workouts. And all of that is a CRUD app, which is create, read, update, delete, all right? And on the topic of CRUD, just for a second, I've noticed, because on Twitter and looking at other stuff online, that maybe CRUD has this reputation of being an older style of developing apps, right?
[00:05:35]
CRUD apps are out-of-date compared to RPC design applications, and I just wanna say that is so far from the truth. So if for any moment, you want to build a CRUD up, or you are hesitant about building a CRUD up, everything that continues to be built is going to implement and be a CRUD app, right?
[00:06:03]
Yes, there's aspects where you can use RPC, right? Something like gRPC is great for microservices, but most apps that we use, like Instagram, Twitter, whatever, literally you can name it, it's going to have CRUD aspects to it. So really, do not focus on what the hot, new, sexy thing is, right?
[00:06:25]
At the end of the day, the foundation is gonna get you a lot further, in my opinion, of how things are written. And even at Twitch, a lot of the work that's done, all the applications are CRUD apps at the end of the day. So what is the tech stack, right?
[00:06:38]
The back-end is gonna be in Go, if you were wondering what the back-end was going to be at this point, that's pretty funny. Obviously, it's gonna be in Go, that's it, that's the course, right? You will hear a lot of time people talk about Go, people talk about the standard library that comes with Go, kind of the out of box solution when you install Go, what you have right away.
[00:07:03]
A lot of purists will say that you can write everything in Go with no external libraries needed. And I wanna say they are correct, you don't need external libraries in Go, okay? You can build everything from scratch, it's kind of the standard library is so rich that if you have a giant import list in your file, it could be a bit of that's an interesting thing.
[00:07:27]
Cuz in Go, typically, a lot of things are made internally using the standard library. So for something like routing, you could, of course, use the standard library, right? It's actually not too difficult to implement. The reason why I chose to use an external library, and I'll get to the reason why she in particular, is because I thought of in real-world aspects.
[00:07:51]
When someone is working in a team on a project at a company, there's gonna be instances we're gonna be importing external libraries, there's no other way around it, right? They are there for a reason, they're proven, they're battle-tested, and they work. And what they do is they make your job easier, right?
[00:08:10]
Now, not all external libraries will do that, some of them are very opinionated, some of them may take away from the true aspect of what you want to implement. But for me, I want to bring a routing library that I trusted, that I've used before, and one that has a really good rep, and that is Chi.
[00:08:28]
So we're gonna be using Chi for our routing library, and we're also gonna be bringing a lot of other external libraries as well. So not be doing a lot of those, but I'm gonna introduce the libraries that I think a lot of Go developers use on a day-to-day and continue to use on a day-to-day basis.
[00:08:47]
In our database, it's gonna be in Postgres, I've already talked about this, it's going to be spun up using Docker. So we're gonna have a separate Docker container that's gonna have our Postgres database. We're gonna be able to connect to our Docker container that's gonna have Postgres running, right?
[00:09:00]
And then we're gonna use, psql, or whatever kind of UI for database that you have to check out what's happening in that database. I think the cool part about this is we're gonna be building everything from absolute scratch, right? So we're gonna start with an empty directory, I'm gonna create literally touch main.go, to create the file, I'm gonna spin it up.
[00:09:20]
So there's no boilerplate, there's no use this and then pull this in, and there's all this coterie written, and you're what does that do? I personally have a bit of a beef with that style, right? If you wanna teach someone, let's truly teach them, let's do it all from scratch and show them how things are done.
[00:09:39]
And so, I think maybe in some cases, that's easier said than done, but in Go, absolutely, we don't need anything to demonstrate what we're gonna be doing. So just having a computer, the prerequisites I mentioned earlier, will get us from nothing to something at the end.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops