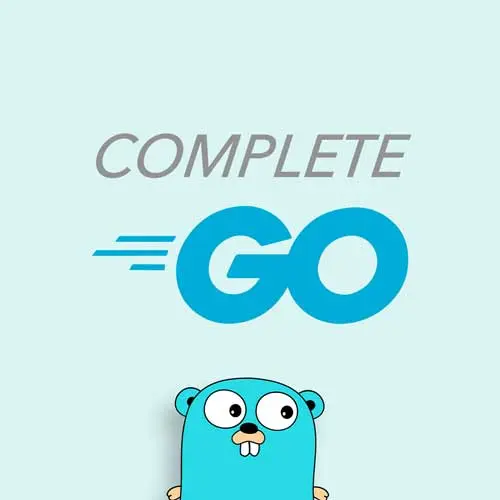
Lesson Description
The "Constants & Enums" Lesson is part of the full, Complete Go for Professional Developers course featured in this preview video. Here's what you'd learn in this lesson:
Melkey demonstrates how to define constants. Unlike other variables, constants will not throw an error for being unused. The iota identifier is also introduced to create enum-like variables with incrementing values.
Transcript from the "Constants & Enums" Lesson
[00:00:00]
>> Melkey: Next we can quickly talk about constants, obviously they don't change. To use and to declare a const, you can just use C-O-N-S-T, and then say something like pi equals like 3.14, all right? Very key distinction between variable and const, you can't re-sign this to anything, it's gonna yell at you.
[00:00:18]
But another cool thing is, the compiler does not care if you have an unused const. You can have a const and you can never reference it again, compilers don't care. But if it's a variable, it does, okay? And the reason for that is actually it's a memory allocation reason, cuz if you have a variable that's declared that can't change values, it wants to make sure it knows what the value is when it's compiling, right?
[00:00:41]
It's gonna use a string, it's unused, it doesn't allocate any memory to it. Or if that string is going to be something that you assign later, the compiler is aware of it versus a const at the time that you write it, the time it compiles, it's gonna know, it's gonna allocate a certain amount of memory for this constant.
[00:00:55]
So it doesn't care if it's unused, cuz you can't change it, right? It takes advantage of that concept. With consts, you can do a few different things. You can do like consts and again, you can put something like Monday = 1, Tuesday = 2, and then Wednesday = 3.
[00:01:15]
So you have this quick way to assign consts with integers or any kinda value you want, you can do format.printf. Again, I'm not gonna print the the pie cuz the compiler doesn't care but even with printf, we can do something like Monday, we can do press module %, Tuesday module %, Wednesday.
[00:01:45]
And then put them in the order they come in. You can see here the compiler gives me a warning that we have these format specifiers, but we haven't specified the type associated to them. So we can just do Monday, Tuesday, and Wednesday, save this. Run it, there you go, Monday, 1, Tuesday, 2, Wednesday, 3, okay?
[00:02:04]
So const have a certain use case. There's also typed versus untyped const, so you can do something like const, typed age, oops! Age, it's gonna be an int. It's gonna directly specify. You can do 25 or you can do const. UntypedAge and just set it to 25 like so.
[00:02:25]
These are gonna be the same thing. So you can do format print line. You can say typedAge = untypedAge. Save this and this should print true, there you go. So it doesn't matter, right? A little bit more flexibility with consts, constants, I should say. With consts, there's a pretty cool thing that we could do.
[00:02:48]
So, a lot of people when they look at Go and they maybe come from a different language, notice that there is a certain lack of features, right? Of course, it's gonna happen. One feature that Go locks that is one that a lot of people would like to see are enums, all right?
[00:03:06]
And so Go does not have a native enum structure or type. However, there is a keyword function that provides a surface level use of an enum. So here we can do const, right? We can open up our parentheses and let's say we're gonna do some consts or enums for months.
[00:03:28]
So we can say Jan and here we can set it to an int, and we can use the built-in keyword iota. And I'm just gonna do plus 1. So the plus 1 just means it's not gonna start at 0, it's gonna start at 1, January being the first month.
[00:03:43]
And now if I do Feb, I can just leave it like this, I can do March and April. And what it's gonna do is enumerate February, March, April as the next integer value from the start of our iota declaration. So if January over here, this is going to be 1, right?
[00:04:02]
Then Feb will be 2, March will be 3, April will be 4, and so forth and so forth. As long as they are declared in the scope of our constants here through the iota scope.
>> Speaker 2: You could admit the int, right?
>> Melkey: Let's see, think so. I guess you can, yeah.
[00:04:25]
Yeah, I think you can maybe specify this definitely, but yeah. You can omit it or not. It's actually validate, that's a format. Let's just do a quick printf Jan. Let's do [INAUDIBLE].
>> Melkey: And April, apr. Okay, we'll put Jan, Feb, March, April. Break the line. Yeah, you can definitely omit it.
[00:05:00]
So that's like a very of an implementation of enums. I kind of suspect that's why the Go team has yet to fully implement enums into the core library, because of the iota. But I do think there's a lot of different use case of enums that go beyond just making sure that a string representation is represented as an integer.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops