The Last Algorithms Course You'll Need
Ace those tough interview questions! Learn big O time complexity, fundamental data structures like arrays, lists, trees, graphs, and maps, and common searching and sorting algorithms.
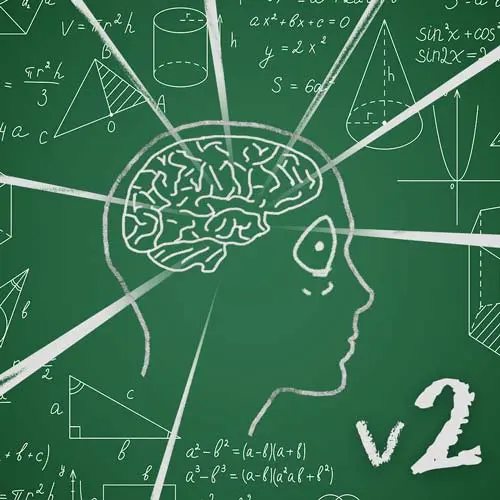
Why Take This Course?
Deepen You Data Structure & Algorithm Understanding and Solve Complex Problems

Build a Strong Foundation

Hands-On Challenges

Ace the Interview

Become a Better Problem Solver

What You'll Learn
Data Structures and Algorithms Every Engineer Should Know
This beginner friendly DSA course will not just go over how to use common algorithms, but how they are implemented and their running times. You will leave this course with a deeper understanding of when and how you can apply these skills in your projects.
- Gain a practical understanding of Big O time complexity.
- Learn common data structures like Arrays, Lists, Queues, Trees, Heaps, Graphs, and Maps.
- Implement searching and sorting algorithms from scratch including, BubbleSort, QuickSort, Linear/Binary Search, Depth/Breadth-First Search, and more!
- Confidently use algorithms and data structures to have successful interviews.
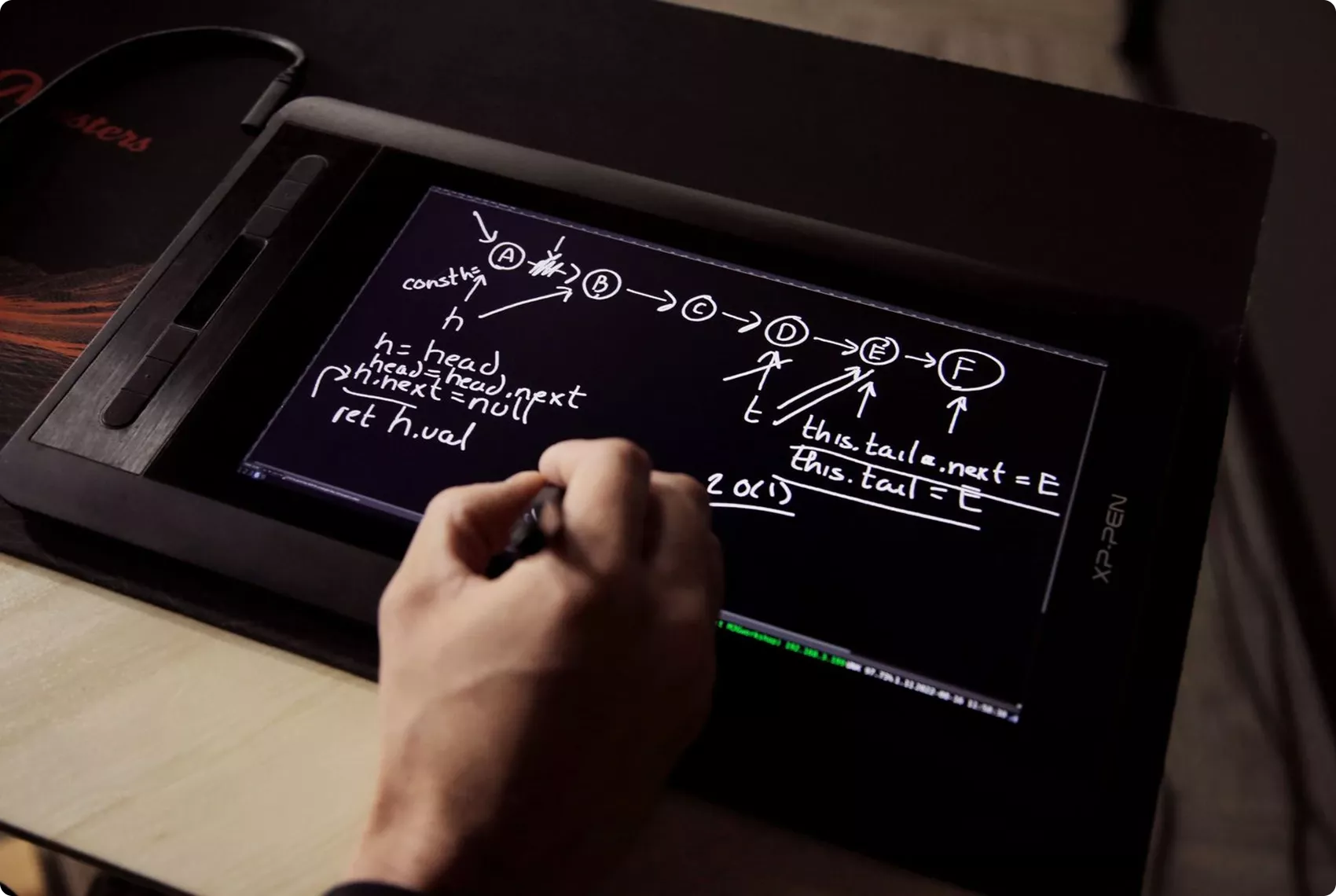
Your (Awesome) Instructor
Programmer, Educator, and Entertainer
Inspiring millions of people to build software and have fun doing it.
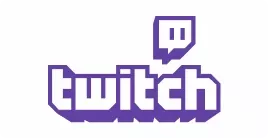
ThePrimeagen on Twitch
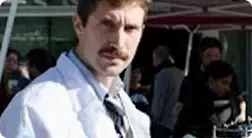
ThePrimeTime
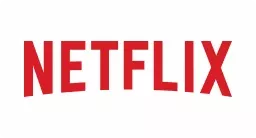
Senior Software Engineer
Coursework
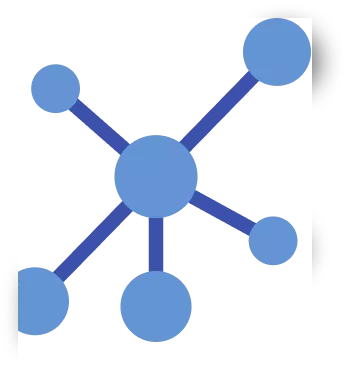
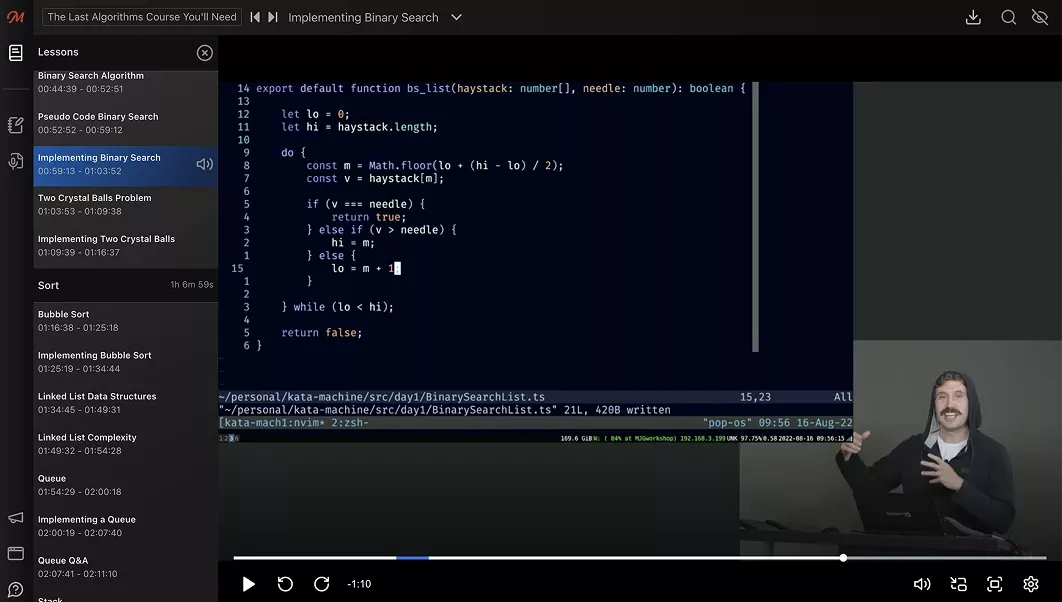
Best in Class Course Player
Data Structure & Algorithm Learning Starts Here
- Course Progress: Learn at your own pace and pick up right where you left off.
- Robust Note-Taking: Take notes alongside transcripts to easily reference information while learning.
- Q&A and Code Corrections: Submit and view questions and answers, as well as code corrections.
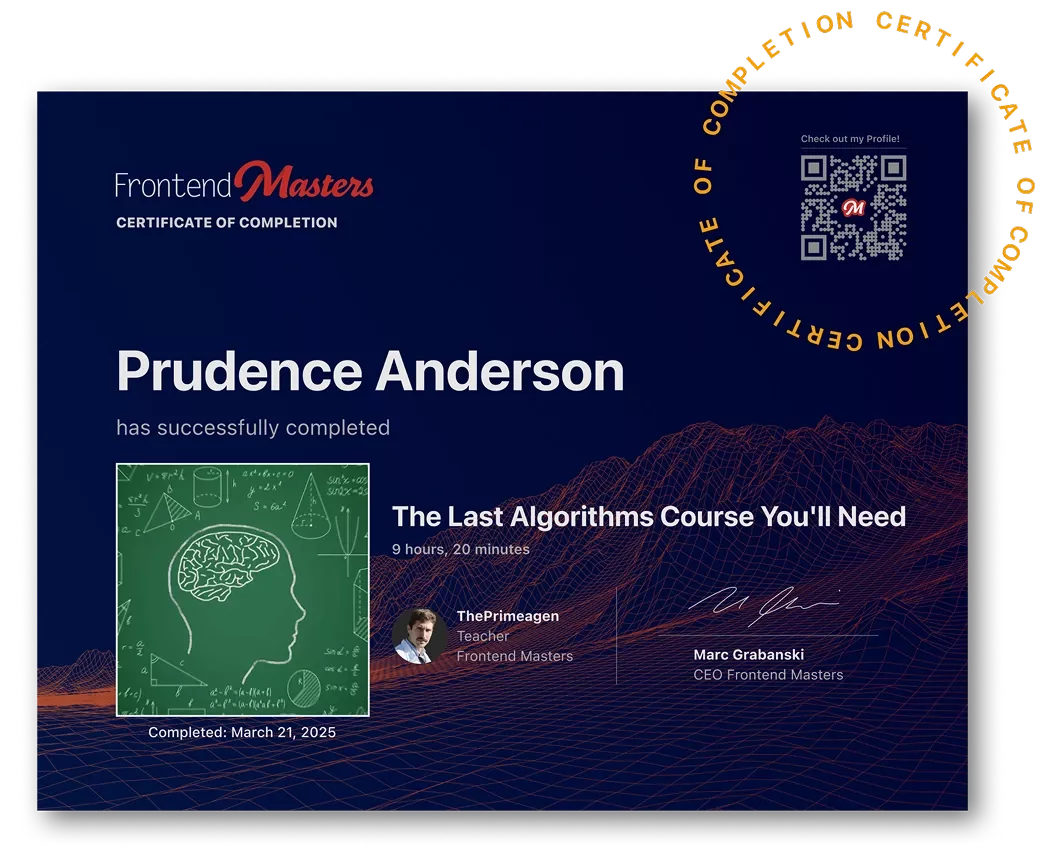
Earn a Completion Certificate
After completing this course, you'll receive a certificate of completion that serves as proof of your achievement, showcasing your expertise, and commitment to professional development. You can easily share this certificate on your LinkedIn profile to highlight your new skills and demonstrate continuous learning to potential employers and professional connections.
Get Started with The Last Algorithms Course You'll Need and Much More
- 200+ In-depth Courses
- 21 Learning Paths
- Industry Leading Experts
- Live Interactive Workshops
What They're Saying about ThePrimeagen
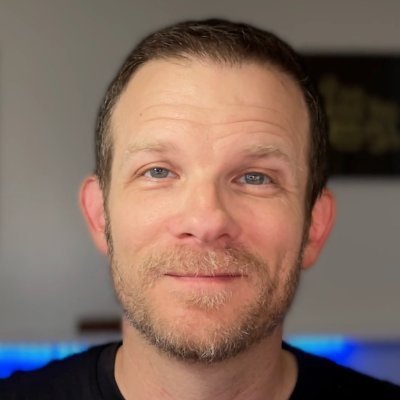
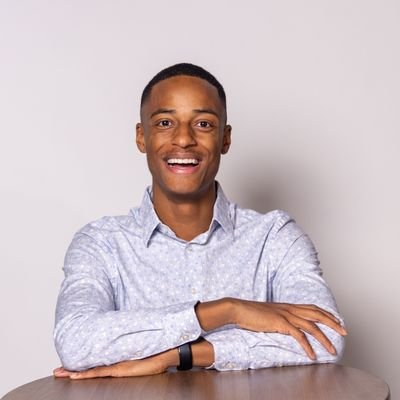
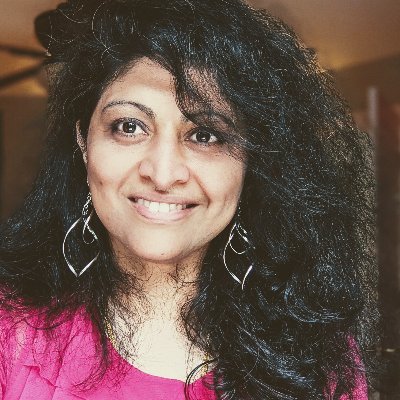
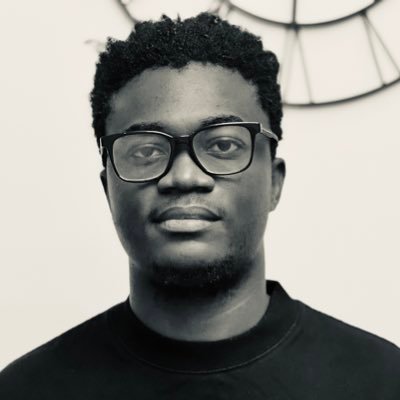
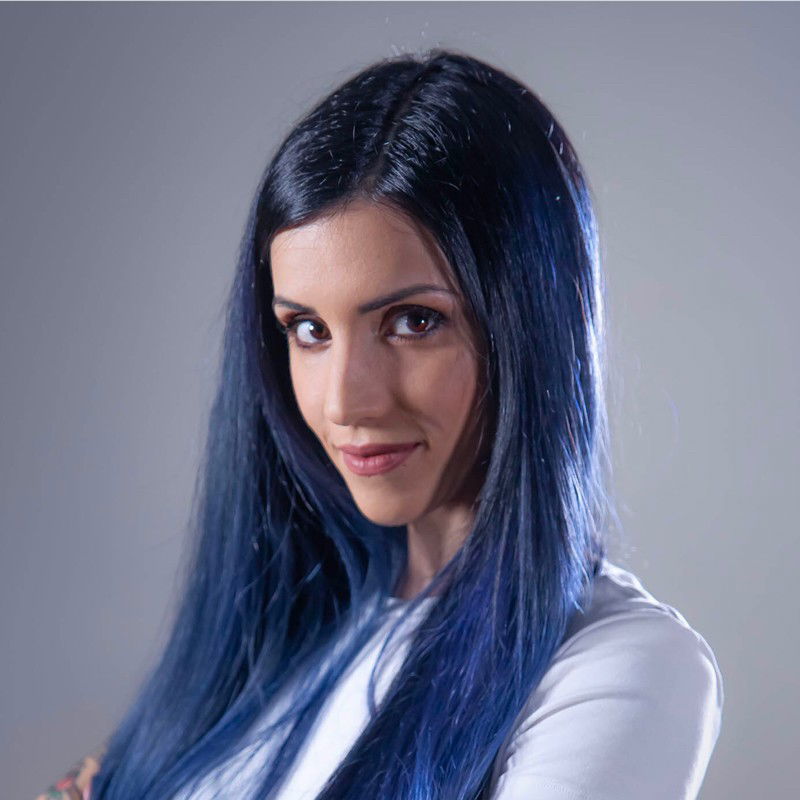
This course is a must for anyone eager to build a strong foundation in algorithms. Whether you're new to the subject or just need a refresher, It provides a clear, methodical learning path that simplifies even the most challenging concepts. I highly recommend it for beginners looking to start their journey into algorithms and computer science.
I just completed this course by The Primeagen on Frontend Masters. This was an incredible course covering big o time complexity, data structures like arrays, lists, trees, graphs, and maps, and searching and sorting algorithms. I feel I have a better grasp on the technical side of backend development and I feel I have progressed as a developer as a whole by taking this course. Thank you, The Primeagen for a wonderful course!
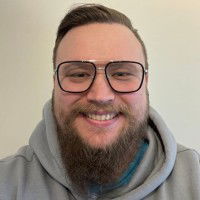