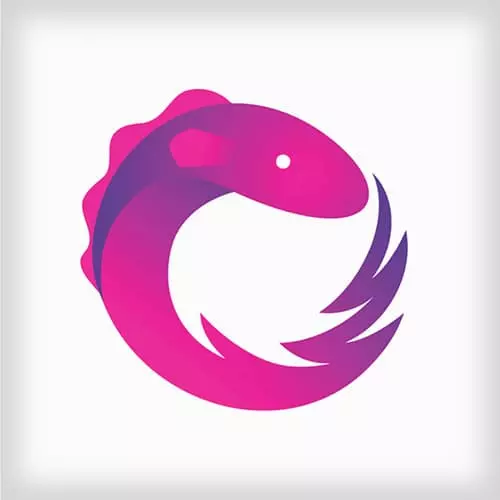
Check out a free preview of the full Advanced Asynchronous JavaScript course
The "Q&A: Time Travel in Redux and Scheduler Class" Lesson is part of the full, Advanced Asynchronous JavaScript course featured in this preview video. Here's what you'd learn in this lesson:
Jafar takes a questions from a students about time travel and redux and scheduler class.
Transcript from the "Q&A: Time Travel in Redux and Scheduler Class" Lesson
[00:00:00]
>> Speaker 1: So to implement time travel with this, could we just use the replay operator?
>> Jafar Husain: You wouldn't use replay for that. What you would do is, so from a time travel perspective, the easiest thing to do might be to just kick off. So there's a variety of things you can do.
[00:00:15]
You can store the previous actions, you can store all the previous states in an array, and then you can just [SOUND] hoop to those states, right. I believe that's how time trouble works in Redux. It just store all the previous, the key, the secret sauce, is that if you notice here I'm not changing the state, I'm creating a new state.
[00:00:33]
I'm not modifying the counter on last state, I'm creating a whole new state object. And because of that what Redux can do is it can literally store every possible state object that's ever been generated by your app, memory allowing, right, and then it can just [SOUND] pop to any part in history and time.
[00:00:53]
And that's one of the cool things about Redux. So that's what he means when he says time travel. Cuz you never end up modifying state, you've always preserved all the old states. In debug mode, Redux can just store all your old states and just [SOUND] hop to them.
[00:01:09]
>> Jafar Husain: Any other questions?
>> Speaker 1: On the chat, someone asked about if you could give a note about RxJS scheduler class?
>> Jafar Husain: Yeah, the scheduler. So a scheduler is a function that decides when data, when code runs. So in general you're not gonna be using schedulers directly for the most part.
[00:01:29]
There are two functions that you use, that typically you use with, to schedule things. So what do I mean when I say decides when code runs? Well let's say I'm making an animation, for example, right, and I wanna make sure that the animation is, I basically only wanna do things on request animation frame.
[00:01:51]
Does that make sense? Right, so there's two things you can do to an observable, rather two times you can schedule something happening with an observable. So I can take an observable, you know what, what the heck, let's write it.
>> Jafar Husain: There's two interesting methods. One is observeOn(scheduler) and SubscribeOn.
[00:02:20]
So there's two times things happen with an observable. One is when you subscribe. So if you subscribe to an observable, one thing that can happen is that that subscription happens right away. You can also schedule a subscription to occur. So when I say schedule I mean, let's say I don't want the subscription to occur for 500 milliseconds.
[00:02:37]
In other words, I want a set time out and then actually cause the subscription to occur. That's when I can use a scheduler and I can call subscribe on. The other thing that I can do is when I get a notification, an ie next complete an error, rather than getting them as soon as the producer sends them.
[00:02:54]
I can say, you know what, schedule these notifications so they don't happen, say, until the next tick or on the set time out. Does that make sense? So those are two times where you can introduce concurrency, basically decide when you get called inside of a function. So let's see how observeOn would work.
[00:03:22]
>> Jafar Husain: So you call observeOn on an observable.
>> Jafar Husain: So we have this observable, and whenever the observable sends a message to us, instead of forwarding it on to the observer, we're actually gonna schedule it to happen later. So I'm gonna go, it's the same thing we've been doing up till now, I'm gonna subscribe to self.
[00:03:57]
>> Jafar Husain: So if we just do this we would be accomplishing nothing, we'd just be sending the observer the next method.
>> Jafar Husain: By the way, a scheduler is nothing fancy, it is literally just like a subscription or an observable. It's a function that's been wrapped up to look like an object.
[00:04:15]
Does that make sense? So it's just a function you call. The scheduler I'll use in this will literally just be a function. It's a little more complicated in RxJS, but the basic idea is here.
>> Jafar Husain: All right, so if we did this we basically no opt, right. All I'm doing is I'm just forwarding stuff along.
[00:04:41]
But if I do this,
>> Jafar Husain: Right, now does anybody kinda get a sense of what a scheduler does? Let's see if we can use observeOn to ensure that an observable doesn't call us for a few seconds.
>> Jafar Husain: So I'm gonna create, see, you know what, we never implemented the simplest constructor for an observer, which is just of.
[00:05:34]
Do that real quick cuz it will be easier. So remember of just takes a value, it actually takes multiple values, but for this purpose for me to keep things simple.
>> Jafar Husain: And it's just gonna turn around and next that value to the observer.
>> Jafar Husain: Now notice I'm just gonna no op in the unsubscribe here because I've already delivered the two values before I've even handed in the subscription.
[00:06:11]
And that's one thing to understand, that observable can be synchronous, it just it doesn't always mean it's asynchronous. It's always about push, it's not about asynchrony.
>> Jafar Husain: When you're pushing in Java script, because it's single threaded, you can be asynchronous if you want to, but you can also just do everything synchronously.
[00:06:31]
So I can fire hose data at somebody when they subscribe as soon as they subscribe. So now that I've built myself a static factory function for an observable that just onNext an individual value, let's see what happens when we subscribe to that.
>> Jafar Husain: Did I call next, or complete?
[00:06:54]
Does anybody remember?
>> Speaker 1: Did an of.
>> Jafar Husain: Yeah.
>> Speaker 1: Yes.
>> Jafar Husain: Great.
>> Speaker 1: But on line 81, it said subscribe instead of unsubscribe.
>> Jafar Husain: Line 81? Thank you.
>> Jafar Husain: Right, everybody understands and expects that? But let's say I don't wanna get this message for five seconds.
>> Jafar Husain: Try and get that on one line.
[00:07:58]
>> Jafar Husain: So I've passed in a scheduler, and as we can see a scheduler's just a glorified function that accepts some action, and then executes it probably later on, right. In this case I'm just gonna execute it in five seconds. And so if we run,
>> Jafar Husain: Should have picked a shorter time,
[00:08:20]
>> Jafar Husain: Right, all I did was instead of doing the thing I wanted to do, I created a function which will do the thing I wanted to do, and then I handed it to a scheduler, which is nothing but a function that accepts an action, and then the scheduler decides when it runs.
[00:08:36]
I'm not gonna bother defining subscribeOn, you guys kinda get the gist of it. The idea there is that you provide a scheduler that, and then you basically provide the logic behind subscribe inside of a function. You hand it to the scheduler and the scheduler decides when subscribe applies.
[00:08:49]
Why would I wanna use this? Well I'd want to use it if I wanted to make sure that certain actions happened at certain times. A great example might be something like request animation frame. So if I wanted to make sure that an action happened on request animation frame, that I would say only wrote to the canvas on request animation frame.
[00:09:06]
I could observe an observable on a request animation frame scheduler, and then I'm basically gonna wait to update the canvas until request animation frame is called. Does that make sense?
>> Jafar Husain: And that's 90% of what you need to understand about schedulers. They're a little bit more complicated than an object with a couple more methods, but that's the basic idea.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops